
Objective-C: Ruby Look
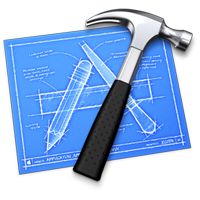
Intro
Prior to learning Objective-C, I programmed first in PHP, then in Python and Ruby. I liked Ruby the most. I liked its simplicity, conciseness, but at the same time and power. And about a week ago, I finally managed to get a hackintosh on my computer (now I have a Macbook Early 2008 Black). It turned out to be OS X Lion Golden Master. I knew that applications for poppies and iPhones write in Objective-C, I even tried to learn it, but without OS X it was unpleasant, perhaps. After installing Xcode 4.2 (I’m already a registered iOS developer), I wrote some very simple console applications. And every time I followed a tutorial, or just tried to write code myself, it occurred to me that, it turns out, Ruby and Objective-C have a lot in common (although this is logical, since both languages were made under the influenceSmalltalk ), despite the fact that the two languages have completely different purposes.

1. Dynamic
We all know that Ruby is a dynamic programming language. But what about Objective-C? Is this just an add-on over C? Not really. It turns out that Objective-C is also a somewhat dynamic language. In it, many things (for example, sending a message) and decisions are made directly at launch time, and not during compilation.
2. The object model
In Ruby, everything is an object. For Objective-C, this is not always true. Since it is an add-on over C, basic data types (such as char, float, double, int, struct ) are not objects. However, the Foundation framework presents functional wrappers above them, as well as seemingly familiar things like strings, mutable arrays, hashes, etc.
3. Sending messages
In Ruby, when we call an object method, an implicit message is sent:
object.send :method, args
that is, the method call is converted to sending the message. In Objective-C, something similar happens:
[object method];
This is just sending a message, not a method call, because if you call a non-existent method, we will not get any errors at the compilation stage, only at runtime (which proves the dynamism of the language). At compile time, it is
[object method]
converted to a call to a C-th function objc_sendMsg(id object, SEL selector)
. As farcaller suggests in the comments:
clang3 on non-existent methods can give compilation errors
By the way, in C ++ language terminology, the methods in Ruby and Objective-C are virtual .
4. Lax typing
Every hacker knows that with Ruby he doesn't need to care about variable types. But not everyone knows that, in principle, Objective-C does too. If we don’t know the type of the object, in Objective-C we can simply specify the type of the object id, which means that the variable can take any type of value. Indeed, id is a pointer to an arbitrary object.
However, for C types, this does not work, only for Objective-C objects. Although resorting to types of C may be required infrequently, because many types of C have implementations on Objective-C - NSNumber, NSString, NSArray, etc.
5. Class declarations, object creation, and properties
In Ruby we can simply define into a class, in Objective-C we are forced to define the description and the implementation of the class itself. Typically, the description and implementation are located in different files. In Ruby, we can define any object variable from any method, in Objective-C we must define in advance all the variables used inside the class.
In principle, objects are created quite easily in both languages. In Ruby, you can simply send a message to the class
new
myObject = MyClass.new
in Objective-C, you first need to allocate memory (
alloc
) for the object , and then initialize it ( init
, initWith…
)MyClass * myObject = [[MyClass alloc] init];
To access instance variables, we must define two methods - getter and setter. In Ruby we can just write
attr_accessor :var
, in Objective-C we have to write type * variable
in the section describing the variables of the class, @property(retain) type * variable;
after the description of the methods, and @synthesize variable;
in the implementation of the class. A small digression: since Objective-C is an add-on over C, we are forced to take care of memory as well. The LLVM compiler supports one interesting feature - Automatic Reference Counting , which takes care of freeing up memory. Xcode version 4.2 and higher uses the LLVM compiler by default, that is, you don’t have to worry about memory management, although, in principle, to fully understand the language, it would be nice to understand such a not-so-simple topic like memory management.
6. Protocols and mixins
In Ruby, we can define modules and then mix them into classes, so something like multiple inheritance is implemented. Objective-C doesn’t specifically. But there are protocols in it. As Wikipedia says :
A protocol is simply a list of method descriptions. An object implements a protocol if it contains implementations of all the methods described in the protocol. Protocols are convenient in that they allow you to highlight the common features of heterogeneous objects and transmit information about objects of previously unknown classes.
That is, for example, a method can receive objects that implement the Serializable protocol and be sure that the object is responding to the message
serialize
.7. Categories and extension of classes
If we want to extend the class, in Ruby we can simply write:
class String
def my_super_method
“New string”
end
end
Thus, we add a method to the class
my_super_method
(although this is not the only way). In Objective-C, we can do something similar using categories:@interface NSString (HelloWorld)
+ (NSString *)helloWorld;
@end
@implementation NSString (HelloWorld)
+ (NSString *)helloWorld
{
return @”Hello, World!”;
}
@end
Categories are usually saved in the ExtensibleClass + NameCategory files - NSString + HelloWorld.m and NSString + HelloWorld.h, and then imported.
Here are just some of the things that made me easier to understand Objective-C. I hope you were interested. Good luck