
Hacking C # CAPTCHA on C # - It's Easy!
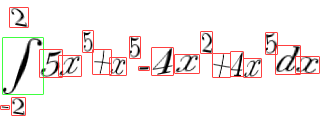
After reading the author’s article about this wonderful captcha, I wanted to write a program to recognize it, as they say just for fun;)
Let's start with the standard preparatory procedure, namely: search for vulnerabilities. Vulnerabilities marked in italics are not used in recognition.
Weaknesses:
- Black characters on a white background.
- No noise and other artifacts (such as lines).
- Symbols never intersect.
- Always the same font.
- There are always 4 terms under the integral.
- Degrees and factors consist of one digit.
- Degrees and factors range from 2 to 5.
- The presence of nonlinear distortion.
- Possible absence of degree or factor x.
- Sometimes, dx stick together in one character.
- The width of the captcha changes.
- Get a captcha bitmap.
- Number all characters.
- Find the coordinates of the upper and lower limits.
- Recognize the constituent characters using a neural network.
- Find the first character of the integrand.
- Recognizing the characters alternately, move right to the last character.
- Solve the resulting integral.
Original captcha

Bitmap
For a unit, we consider a pixel whose brightness according to the HSB color model <0.8, for zero, respectively> = = 0.8. After that, captcha will take the following form:
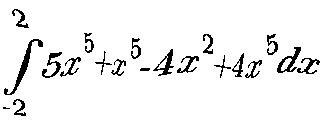
Character numbering
In order to number all the characters, we use the simplest recursive Flood fill algorithm to select connected areas in 8 directions.
public int FloodFill(ref int[,] source, int num, int x, int y)
{
if (source[x, y] == -1)
{
source[x, y] = num;
FloodFill(ref source, num, x - 1, y - 1);
FloodFill(ref source, num, x - 1, y);
FloodFill(ref source, num, x - 1, y + 1);
FloodFill(ref source, num, x, y - 1);
FloodFill(ref source, num, x, y + 1);
FloodFill(ref source, num, x + 1, y - 1);
FloodFill(ref source, num, x + 1, y);
FloodFill(ref source, num, x + 1, y + 1);
return ++num;
}
return num;
}
...
int num = 1;
for (int x = 0; x < CaptchaWidth; x++)
for (int y = 0; y < CaptchaHeight; y++)
num = FloodFill(ref bit, num, x, y);
Character recognition
Given the non-linear distortions and the different size of the characters, the best option for recognizing them is an artificial neural network. To make the task a little easier, I used the free Fast Artificial Neural Network Library . It is written in C ++, and is good because it has interfaces for almost all popular programming languages, including C # .
All characters are reduced to the same size before recognition: in this case 16px x 21px.
Thus, an array of 336 elements (16 * 21) will be fed to the input of the neural network. Each element sets the color of the corresponding pixel as an integer: 0 or 1.
The middle layer consists of 130 neurons. And at the output, an array of 14 elements with real values from 0 to 1, corresponding to numbers from 0 to 9, the sign +, d, x, and stuck dx.
The training took place on 3090 samples, among which the most characters are “x” and least “d”. Despite this, the training process on my C2D e6750 took only 40 seconds.
Code for training NA:
static void Main(string[] args)
{
NeuralNet net = new NeuralNet();
//Указываем слои нейронной сети
uint[] layers = { 336, 130, 14 };
net.CreateStandardArray(layers);
//Устанавливаем случайные веса
net.RandomizeWeights(-0.1, 0.1);
net.SetLearningRate(0.7f);
//Загружаем данные для обучения НС
TrainingData data = new TrainingData();
data.ReadTrainFromFile("train.tr");
//Обучаем сеть
net.TrainOnData(data, 1000, 0, 0.001f);
//Сохраняем готовую НС в файл
net.Save("skynet.ann");
}
The structure of the text file train.tr:
num_train_data num_input num_output
inputdata seperated by space
outputdata seperated by space
...
inputdata seperated by space
outputdata seperated by space
Examples of characters transmitted for recognition:
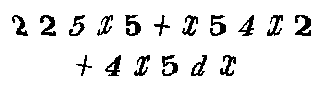
Integral Recognition
First we find and recognize the limits. The upper one can consist of 1-2 digits, with a maximum value of 10. The lower one is in the range from 0 to -10.
Facilitate the task of a neural network:
- If the upper limit consists of two digits, then this is 10 (the lower of three).
- We recognize the minus algorithmically by the threshold value of the symbol height.
- In recognition, we will not take into account the NS outputs that do not match the numbers.
Knowing the number of the integral, the number of objects on the captcha, and the fact that these objects are numbered from left to right, with further sequential recognition of characters, we get approximately the following line “5x5 + x5-4x2 + 4x5dx”. First we simplify the work of the expression parser - we will bring it to the form "+ 5x5 + x5-4x2 + 4x5".
Some patterns make it easy to get the final data to solve the integral:
- The signs "+" and "-" separate the terms.
- The numbers after the "x" are the degree.
- Etc.
Instead of a conclusion
The recognition quality of the entire captcha is impressive: 93.8% in a sample of 500 pieces!
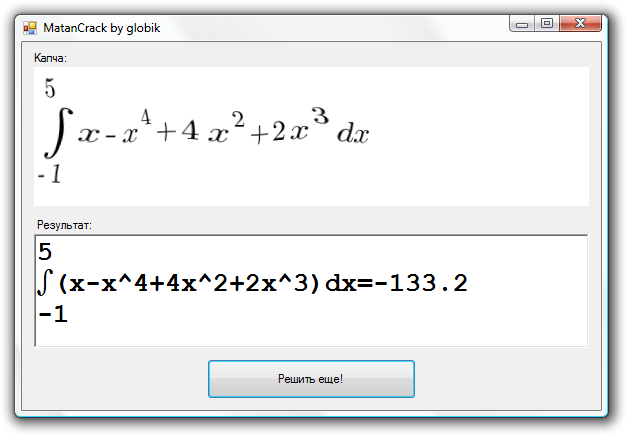
Download the finished program that demonstrates the solution of the integral here . Or along with the source code (+ a program for training a neural network and a training sample) here .
I hope that neither my server nor the server of the grishkaa habrayuzer will fall under the habraeffect;)