PyQt4 - Manage widget layout
- Transfer
An important part of programming is element layout management. Location management is how we place widgets on a form. There are two ways: using absolute positioning or using layout classes.
Absolute positioning
The programmer indicates the position and size of each widget in pixels. When you use the absolute layout, you should understand several things: the
size and position of the widget does not change when the window is resized; the
application may look different on different platforms;
changing the font in your application may ruin the layout;
if you decide to change the layout, you must completely repeat it, which takes away a lot of time We just call the move () method to reposition the widgets, in our case it is QLabel. We arrange them according to the X and Y coordinates. The origin of the coordinate system is in the upper left corner of the window. The X coordinate grows from right to left, and Y grows from top to bottom. Box layout

(I didn’t come up with a translation)
Location management using layout classes is more flexible and practical. This is the preferred way to arrange widgets. Simple layout classes are QHBoxLayout and QVBoxLayout. They arrange widgets horizontally and vertically.
Imagine that we want to place two buttons in the lower right corner of the form. To create such a layout, we will use one horizontal and one vertical box. We get the necessary space by adding a stretch factor. Here we create two QPushButton buttons. Create a horizontal layout and add a stretch factor for both buttons. Create a vertical layout. And at the end, set the main layout for the window. QGridLayout
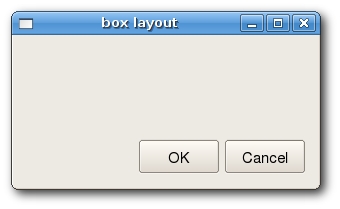
The most universal class of layouts is the layout of the table. This layout divides the space into rows and columns. To create it, the QGridLayout class is used. In our example, we create a button table. We leave one cell empty by adding one QLabel widget. Here we create a table layout. To add a widget to the table, we must call the addWidget () method, passing the widget as well as the row and column numbers as arguments. Widgets can span multiple rows or columns and in the following example we show this. Create a table layout and specify the distance between widgets. If we add a widget to the layout, we can specify how many rows or columns it combines. In our case, reviewEdit concatenates 5 lines.
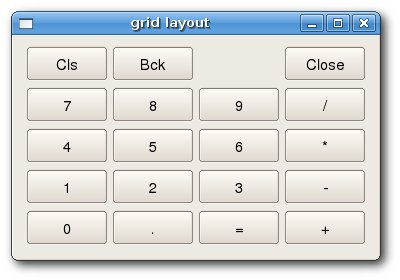

Absolute positioning
The programmer indicates the position and size of each widget in pixels. When you use the absolute layout, you should understand several things: the
size and position of the widget does not change when the window is resized; the
application may look different on different platforms;
changing the font in your application may ruin the layout;
if you decide to change the layout, you must completely repeat it, which takes away a lot of time We just call the move () method to reposition the widgets, in our case it is QLabel. We arrange them according to the X and Y coordinates. The origin of the coordinate system is in the upper left corner of the window. The X coordinate grows from right to left, and Y grows from top to bottom. Box layout
#!/usr/bin/python
import sys
from PyQt4 import QtGui
class Absolute(QtGui.QWidget):
def __init__(self, parent=None):
QtGui.QWidget.__init__(self, parent)
self.setWindowTitle('Communication')
label = QtGui.QLabel('Couldn\'t', self)
label.move(15, 10)
label = QtGui.QLabel('care', self)
label.move(35, 40)
label = QtGui.QLabel('less', self)
label.move(55, 65)
label = QtGui.QLabel('And', self)
label.move(115, 65)
label = QtGui.QLabel('then', self)
label.move(135, 45)
label = QtGui.QLabel('you', self)
label.move(115, 25)
label = QtGui.QLabel('kissed', self)
label.move(145, 10)
label = QtGui.QLabel('me', self)
label.move(215, 10)
self.resize(250, 150)
app = QtGui.QApplication(sys.argv)
qb = Absolute()
qb.show()
sys.exit(app.exec_())

(I didn’t come up with a translation)
Location management using layout classes is more flexible and practical. This is the preferred way to arrange widgets. Simple layout classes are QHBoxLayout and QVBoxLayout. They arrange widgets horizontally and vertically.
Imagine that we want to place two buttons in the lower right corner of the form. To create such a layout, we will use one horizontal and one vertical box. We get the necessary space by adding a stretch factor. Here we create two QPushButton buttons. Create a horizontal layout and add a stretch factor for both buttons. Create a vertical layout. And at the end, set the main layout for the window. QGridLayout
#!/usr/bin/python
import sys
from PyQt4 import QtGui
class BoxLayout(QtGui.QWidget):
def __init__(self, parent=None):
QtGui.QWidget.__init__(self, parent)
self.setWindowTitle('box layout')
ok = QtGui.QPushButton("OK")
cancel = QtGui.QPushButton("Cancel")
hbox = QtGui.QHBoxLayout()
hbox.addStretch(1)
hbox.addWidget(ok)
hbox.addWidget(cancel)
vbox = QtGui.QVBoxLayout()
vbox.addStretch(1)
vbox.addLayout(hbox)
self.setLayout(vbox)
self.resize(300, 150)
app = QtGui.QApplication(sys.argv)
qb = BoxLayout()
qb.show()
sys.exit(app.exec_())
ok = QtGui.QPushButton("OK")
cancel = QtGui.QPushButton("Cancel")
hbox = QtGui.QHBoxLayout()
hbox.addStretch(1)
hbox.addWidget(ok)
hbox.addWidget(cancel)
vbox = QtGui.QVBoxLayout()
vbox.addStretch(1)
vbox.addLayout(hbox)
self.setLayout(vbox)
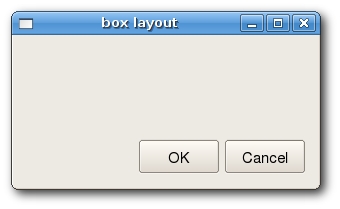
The most universal class of layouts is the layout of the table. This layout divides the space into rows and columns. To create it, the QGridLayout class is used. In our example, we create a button table. We leave one cell empty by adding one QLabel widget. Here we create a table layout. To add a widget to the table, we must call the addWidget () method, passing the widget as well as the row and column numbers as arguments. Widgets can span multiple rows or columns and in the following example we show this. Create a table layout and specify the distance between widgets. If we add a widget to the layout, we can specify how many rows or columns it combines. In our case, reviewEdit concatenates 5 lines.
#!/usr/bin/python
import sys
from PyQt4 import QtGui
class GridLayout(QtGui.QWidget):
def __init__(self, parent=None):
QtGui.QWidget.__init__(self, parent)
self.setWindowTitle('grid layout')
names = ['Cls', 'Bck', '', 'Close', '7', '8', '9', '/',
'4', '5', '6', '*', '1', '2', '3', '-',
'0', '.', '=', '+']
grid = QtGui.QGridLayout()
j = 0
pos = [(0, 0), (0, 1), (0, 2), (0, 3),
(1, 0), (1, 1), (1, 2), (1, 3),
(2, 0), (2, 1), (2, 2), (2, 3),
(3, 0), (3, 1), (3, 2), (3, 3 ),
(4, 0), (4, 1), (4, 2), (4, 3)]
for i in names:
button = QtGui.QPushButton(i)
if j == 2:
grid.addWidget(QtGui.QLabel(''), 0, 2)
else: grid.addWidget(button, pos[j][0], pos[j][1])
j = j + 1
self.setLayout(grid)
app = QtGui.QApplication(sys.argv)
qb = GridLayout()
qb.show()
sys.exit(app.exec_())
grid = QtGui.QGridLayout()
if j == 2:
grid.addWidget(QtGui.QLabel(''), 0, 2)
else:
grid.addWidget(button, pos[j][0], pos[j][1])
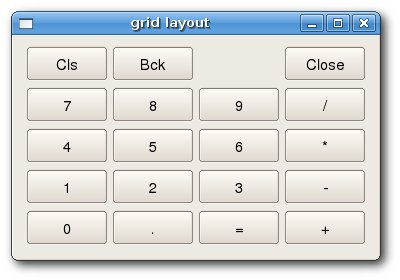
#!/usr/bin/python
import sys
from PyQt4 import QtGui
class GridLayout2(QtGui.QWidget):
def __init__(self, parent=None):
QtGui.QWidget.__init__(self, parent)
self.setWindowTitle('grid layout')
title = QtGui.QLabel('Title')
author = QtGui.QLabel('Author')
review = QtGui.QLabel('Review')
titleEdit = QtGui.QLineEdit()
authorEdit = QtGui.QLineEdit()
reviewEdit = QtGui.QTextEdit()
grid = QtGui.QGridLayout()
grid.setSpacing(10)
grid.addWidget(title, 1, 0)
grid.addWidget(titleEdit, 1, 1)
grid.addWidget(author, 2, 0)
grid.addWidget(authorEdit, 2, 1)
grid.addWidget(review, 3, 0)
grid.addWidget(reviewEdit, 3, 1, 5, 1)
self.setLayout(grid)
self.resize(350, 300)
app = QtGui.QApplication(sys.argv)
qb = GridLayout2()
qb.show()
sys.exit(app.exec_())
grid = QtGui.QGridLayout()
grid.setSpacing(10)
grid.addWidget(reviewEdit, 3, 1, 5, 1)
