React tutorial, part 16: fourth stage of work on a TODO application, event handling
- Transfer
- Tutorial
In today's part of the translation of the React course, we will continue to work on the Todo application and talk about how React handles events.
→ Part 1: course overview, reasons for the popularity of React, ReactDOM and JSX
→ Part 2: functional components
→ Part 3: component files, project structure
→ Part 4: parent and child components
→ Part 5: starting work on a TODO application, basics of styling
→ Part 6: about some features of the course, JSX and JavaScript
→ Part 7: inline styles
→ Part 8: continued work on a TODO application, familiarity with the properties of components
→
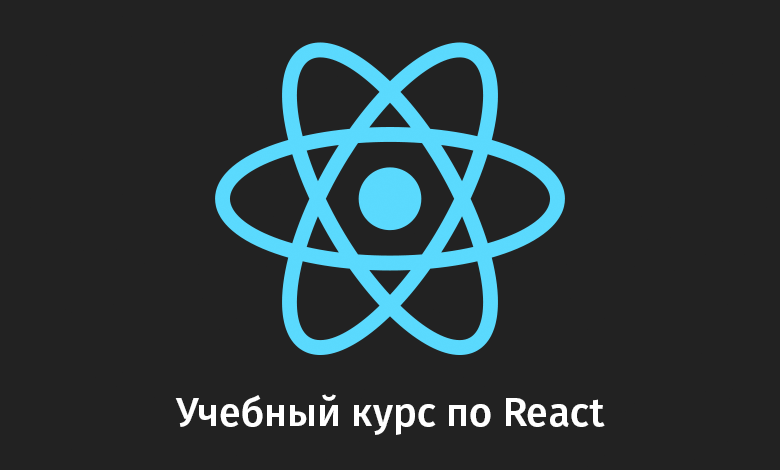
Part 9: properties of components
→ Part 10: workshop on working with properties of components and stylization
→ Part 11: dynamic markup generation and the array method map
→ Part 12: workshop, third stage of working on a TODO application
→ Part 13: class-based components
→ Part 14: a workshop on class-based components, the state of components
→ Part 15: workshops on working with the state of components
→ Part 16: the fourth stage of working on a TODO application, event handling
→ Original
Last time we loaded the to-do list for the application from a JSON file, and then, walking through the resulting array, we formed, using the method
The task today is to transform a component
Recall the component code already existing in our project
In order to be able to modify the data from the to-do list, we need to ensure that what is now stored in
Solving this problem, we must first convert the functional component
It should be noted that after all these transformations, the appearance of the application has not changed, but after completing them, we prepared it for further work on it.
→ Original
Event handling is what drives web applications and distinguishes them from simple static websites. Event handling in React is pretty simple, it is very similar to how events are processed in plain HTML. For example, in React there are event handlers
Here we will consider examples experimenting with a standard application created by tools
This is what our application looks like in a browser.

Application page in the browser
Before we can seriously talk about modifying the state of components using the method
Event handling in React is actually quite simple. If you are familiar with the standard HTML mechanisms used to assign controls to event handlers, like an event handler
For example, in order for HTML tools to make it so that by clicking on a button, a function is executed, you can use this construction (provided that this function exists and is available):
In React, as already mentioned, event handlers are named according to the rules of the camel style, that is, it
Let's work with our code now and make the button react to clicks on it. Instead of passing code to the handler to call the function as a string, we pass the name of the function in curly braces. The preparation of the corresponding fragment of our code will now look like this:
If you look at the component code
Now when you press the button, the text gets into the console
The same effect can be achieved by declaring an independent function and bringing the code of the component file to the following form:
For a complete list of events supported by React, have a look at this documentation page.
Now try to equip our application with a new opportunity. Namely - make it so that when you hover the mouse over the image in the console would be displayed some message. To do this, you need to find a suitable event in the documentation and organize its processing.
In fact, this problem can be solved in different ways, we will demonstrate its solution based on the event
Event handling gives the programmer tremendous opportunities, which, of course, are not limited to displaying messages in the console. Later we will talk about how event processing, combined with the ability to change the state of components, will allow our applications to solve the tasks assigned to them.
As usual - we recommend you take some time to experiment with what you learned today.
Today you have done a little practical work that laid the foundation for major changes to the Todo application, and got acquainted with the mechanisms for handling events in React. Next time you will be offered another workshop and a new topic will be introduced.
Dear readers! If you, having familiarized yourself with the methods of handling events in React, have experimented with what you have learned, we ask you to tell about it.

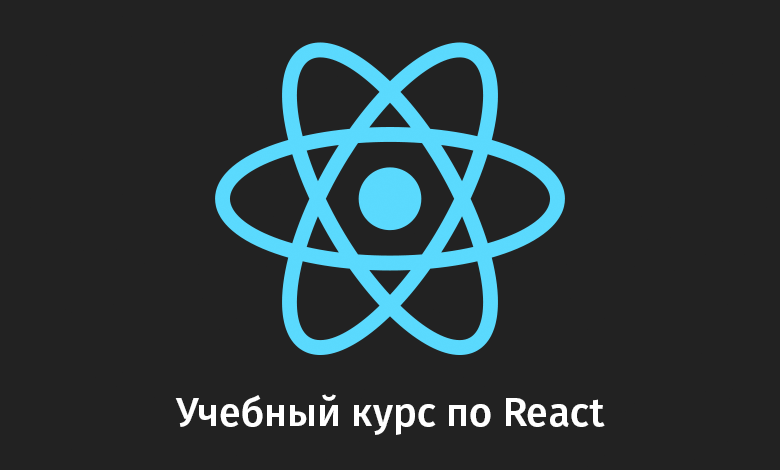
Part 9: properties of components
→ Part 10: workshop on working with properties of components and stylization
→ Part 11: dynamic markup generation and the array method map
→ Part 12: workshop, third stage of working on a TODO application
→ Part 13: class-based components
→ Part 14: a workshop on class-based components, the state of components
→ Part 15: workshops on working with the state of components
→ Part 16: the fourth stage of working on a TODO application, event handling
Session 29. Workshop. TODO application. Stage 4
→ Original
▍Job
Last time we loaded the to-do list for the application from a JSON file, and then, walking through the resulting array, we formed, using the method
map()
, a set of components. We would like to modify this data. And we can only do this if we pre-load them into the component state. The task today is to transform a component
App
into a stateful component and load the imported case data into the state of that component.▍Decision
Recall the component code already existing in our project
App
:import React from"react"import TodoItem from"./TodoItem"import todosData from"./todosData"functionApp() {
const todoItems = todosData.map(item => <TodoItem key={item.id} item={item}/>)
return (
<divclassName="todo-list">
{todoItems}
</div>
)
}
exportdefault App
In order to be able to modify the data from the to-do list, we need to ensure that what is now stored in
todosData
is placed in the component state App
. Solving this problem, we must first convert the functional component
App
to a component based on the class. Then we need to load the data from todosData
to the state and, forming the list of components TodoItem
, bypass the array todosData
, but the array with the same data, which is stored in the state. Here is what it will look like:import React from"react"import TodoItem from"./TodoItem"import todosData from"./todosData"classAppextendsReact.Component{
constructor() {
super()
this.state = {
todos: todosData
}
}
render() {
const todoItems = this.state.todos.map(item => <TodoItem key={item.id} item={item}/>)
return (
<divclassName="todo-list">
{todoItems}
</div>
)
}
}
exportdefault App
It should be noted that after all these transformations, the appearance of the application has not changed, but after completing them, we prepared it for further work on it.
Lesson 30: Handling React Events
→ Original
Event handling is what drives web applications and distinguishes them from simple static websites. Event handling in React is pretty simple, it is very similar to how events are processed in plain HTML. For example, in React there are event handlers
onClick
and onSubmit
that are similar to similar HTML mechanisms presented in the form onclick
and onsubmit
, not only in terms of names (in React, however, their names are formed using camel style), but also exactly work with them. Here we will consider examples experimenting with a standard application created by tools
create-react-app
, the component file of App
which contains the following code:import React from"react"functionApp() {
return (
<div>
<imgsrc="https://www.fillmurray.com/200/100"/>
<br />
<br />
<button>Click me</button>
</div>
)
}
export default App
This is what our application looks like in a browser.

Application page in the browser
Before we can seriously talk about modifying the state of components using the method
setState()
, we need to deal with events and event handling in React. Event processing mechanisms allow an application user to interact with it. An application can react, for example, to eventsclick
orhover
by performing certain actions when these events occur. Event handling in React is actually quite simple. If you are familiar with the standard HTML mechanisms used to assign controls to event handlers, like an event handler
onclick
, then you will immediately see the similarities with these mechanisms that React offers us.For example, in order for HTML tools to make it so that by clicking on a button, a function is executed, you can use this construction (provided that this function exists and is available):
<button onclick="myFunction()">Click me</button>
In React, as already mentioned, event handlers are named according to the rules of the camel style, that is, it
onclick
will turn into onClick
. The same is true for the event handler onMouseOver
, and for other handlers. The reason for this change is that it uses an approach to naming entities, common to JavaScript. Let's work with our code now and make the button react to clicks on it. Instead of passing code to the handler to call the function as a string, we pass the name of the function in curly braces. The preparation of the corresponding fragment of our code will now look like this:
<button onClick={}>Click me</button>
If you look at the component code
App
that we use in this example, you will notice that there is not yet declared a function that you plan to call when you press a button. In general, right now we can easily get away with an anonymous function declared directly in the code describing the button. Here is what it will look like:<button onClick={() => console.log("I was clicked!")}>Click me</button>
Now when you press the button, the text gets into the console
I was clicked!
. The same effect can be achieved by declaring an independent function and bringing the code of the component file to the following form:
import React from"react"functionhandleClick() {
console.log("I was clicked")
}
functionApp() {
return (
<div>
<imgsrc="https://www.fillmurray.com/200/100"/>
<br />
<br />
<buttononClick={handleClick}>Click me</button>
</div>
)
}
export default App
For a complete list of events supported by React, have a look at this documentation page.
Now try to equip our application with a new opportunity. Namely - make it so that when you hover the mouse over the image in the console would be displayed some message. To do this, you need to find a suitable event in the documentation and organize its processing.
In fact, this problem can be solved in different ways, we will demonstrate its solution based on the event
onMouseOver
. When this event occurs, we will display a message in the console. Here is what our code will now look like:import React from"react"functionhandleClick() {
console.log("I was clicked")
}
functionApp() {
return (
<div>
<imgonMouseOver={() => console.log("Hovered!")} src="https://www.fillmurray.com/200/100"/>
<br />
<br />
<buttononClick={handleClick}>Click me</button>
</div>
)
}
export default App
Event handling gives the programmer tremendous opportunities, which, of course, are not limited to displaying messages in the console. Later we will talk about how event processing, combined with the ability to change the state of components, will allow our applications to solve the tasks assigned to them.
As usual - we recommend you take some time to experiment with what you learned today.
Results
Today you have done a little practical work that laid the foundation for major changes to the Todo application, and got acquainted with the mechanisms for handling events in React. Next time you will be offered another workshop and a new topic will be introduced.
Dear readers! If you, having familiarized yourself with the methods of handling events in React, have experimented with what you have learned, we ask you to tell about it.
