
JavaScript: 7 useful little things
- Transfer
The author of the note, the translation of which we are publishing today, says that in JavaScript, as in any other programming language, you can find many small tricks designed to solve a variety of problems, both simple and quite complex. Some of these techniques are widely known, while others, not so common, may pleasantly surprise those who do not know about them. Now we will look at 7 useful JavaScript programming techniques.
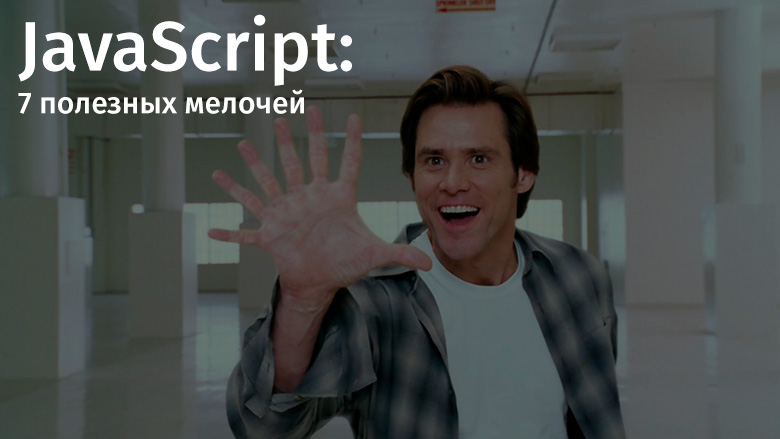
In JavaScript, generating an array containing only unique values from another array is probably easier than you think:
I like the way this problem can be solved by jointly using the operator
Have you ever needed to remove values from an array that can be converted to a logical type
As you can see, in order to get rid of all such values, it is enough to pass
I am sure that you can create an object that seems empty, using the object literal syntax:
An object created in this way does not have properties and methods that are not added to it by the programmer.
Those who write in JavaScript, has always been a need to create such objects, which are included to a content of other sites. This task became especially urgent when classes appeared in JavaScript, then when programmers had to work with something like programmatic representations of widgets. Here's how to create a new object based on several other objects:
The operator
Setting default function argument values is a great extension to JavaScript. But here's how to make it so that without passing some required parameters the functions would simply refuse to work:
Before us is an additional level of verification of what is passed to functions.
Destructuring is a new useful JavaScript feature, but sometimes properties retrieved from objects need to be assigned names that are different from those they have in those objects. Here's how to do it:
This technique is useful in cases where you need to avoid a conflict of variable names or constants.
For many years, we have written regular expressions to parse query strings, but these times have passed. Now, to solve this problem, you can use the wonderful API URLSearchParams :
Using the API is
Modern JavaScript is evolving very quickly, with various useful improvements constantly appearing in it. But improving the language does not mean that programmers do not need to think about the code and look for effective solutions to various problems that confront them. We hope the little JavaScript tricks we talked about today come in handy.
Dear readers! Do you know any useful JS programming tricks? If so, please share them.

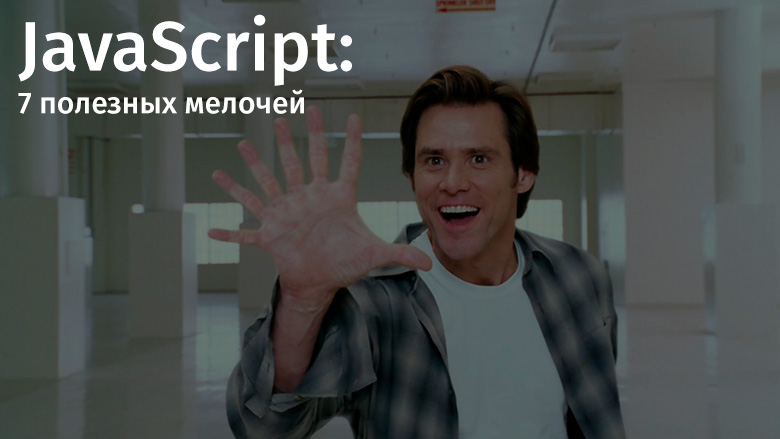
1. Getting unique array values
In JavaScript, generating an array containing only unique values from another array is probably easier than you think:
var j = [...new Set([1, 2, 3, 3])]
// [1, 2, 3]
I like the way this problem can be solved by jointly using the operator
...
and the data type Set
.2. Arrays and logical values
Have you ever needed to remove values from an array that can be converted to a logical type
false
? For example, this values such as 0
, undefined
, null
, false
. You may not have known that in order to do this, you could do this:myArray
.map(item => {
// ...
})
// Избавляемся от ненужных значений
.filter(Boolean);
As you can see, in order to get rid of all such values, it is enough to pass
Boolean
arrays to the method .filter()
.3. Creating truly empty objects
I am sure that you can create an object that seems empty, using the object literal syntax:
{}
. But a prototype ( __proto__
) will be assigned to such an object , it will have a method hasOwnProperty()
and other methods of the objects. In order to create a truly empty object , which, for example, can be used as a “dictionary”, you can do this:let dict = Object.create(null);
// dict.__proto__ === "undefined"
// У объекта нет никаких свойств до тех пор, пока вы их в явном виде не добавите к нему
An object created in this way does not have properties and methods that are not added to it by the programmer.
4. Merging objects
Those who write in JavaScript, has always been a need to create such objects, which are included to a content of other sites. This task became especially urgent when classes appeared in JavaScript, then when programmers had to work with something like programmatic representations of widgets. Here's how to create a new object based on several other objects:
const person = { name: 'David Walsh', gender: 'Male' };
const tools = { computer: 'Mac', editor: 'Atom' };
const attributes = { handsomeness: 'Extreme', hair: 'Brown', eyes: 'Blue' };
const summary = {...person, ...tools, ...attributes};
/*
Object {
"computer": "Mac",
"editor": "Atom",
"eyes": "Blue",
"gender": "Male",
"hair": "Brown",
"handsomeness": "Extreme",
"name": "David Walsh",
}
*/
The operator
...
greatly simplifies the solution of the task of merging objects.5. Required function parameters
Setting default function argument values is a great extension to JavaScript. But here's how to make it so that without passing some required parameters the functions would simply refuse to work:
const isRequired = () => { throw new Error('param is required'); };
const hello = (name = isRequired()) => { console.log(`hello ${name}`) };
// Тут будет выдана ошибка, функции не передан аргумент name
hello();
// Здесь тоже будет ошибка
hello(undefined);
// Эти варианты вызова функции будут работать нормально
hello(null);
hello('David');
Before us is an additional level of verification of what is passed to functions.
6. Destructive assignment and new names of extracted properties of objects
Destructuring is a new useful JavaScript feature, but sometimes properties retrieved from objects need to be assigned names that are different from those they have in those objects. Here's how to do it:
const obj = { x: 1 };
// Теперь мы можем работать с obj.x как с x
const { x } = obj;
// А теперь obj.x для нас выглядит как otherName
const { x: otherName } = obj;
This technique is useful in cases where you need to avoid a conflict of variable names or constants.
7. Parsing query strings
For many years, we have written regular expressions to parse query strings, but these times have passed. Now, to solve this problem, you can use the wonderful API URLSearchParams :
// Предполагается, что мы работаем с "?post=1234&action=edit"
var urlParams = new URLSearchParams(window.location.search);
console.log(urlParams.has('post')); // true
console.log(urlParams.get('action')); // "edit"
console.log(urlParams.getAll('action')); // ["edit"]
console.log(urlParams.toString()); // "?post=1234&action=edit"
console.log(urlParams.append('active', '1')); // "?post=1234&action=edit&active=1"
Using the API is
URLSearchParams
much easier than solving the same problems using regular expressions.Summary
Modern JavaScript is evolving very quickly, with various useful improvements constantly appearing in it. But improving the language does not mean that programmers do not need to think about the code and look for effective solutions to various problems that confront them. We hope the little JavaScript tricks we talked about today come in handy.
Dear readers! Do you know any useful JS programming tricks? If so, please share them.
