AMS and the magic crystal

This time, I propose to do a little magic (why not?) And create a magic crystal for our daily needs. We will use it for its intended purpose - for the divination of various non-obvious entities and events. And we need only two ingredients to make this artifact - Arduino Mega Server and the nooLite SD111-180 wireless LED strip controller .
The crystal will not be decorative (to deceive a gullible public), but the most functional, with many magical properties.
So, let's get started ...
School of Magic
As you yourself understand, you cannot just take and perform some miracle, you must first go through the school of magic and learn the basics of this difficult art. And here I am referring you to a series of articles ( one , two , three ) on the integration of nooLite equipment in home automation systems and recommend that you familiarize yourself with them before further reading. In these articles, all the basics and technical details of how the Arduino Mega Server works with nooLite wireless equipment are detailed. Therefore, I will continue the entire narrative assuming that you have already read the previous articles.
Module
We will conduct all experiments with the nooLite SD111-180 wireless controller, which allows you to control LED strips "over the air", without any wires. Connection, as always with nooLite, the simplest is two wires to the power supply for LED strips and four to the tape itself: plus and three channels R, G, B.
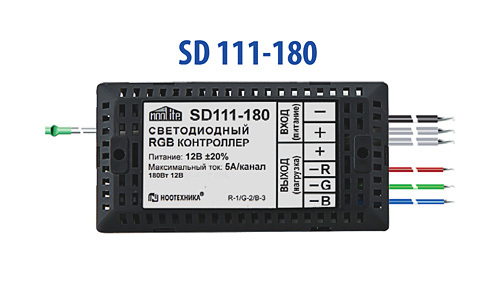
Software part
Previous articles talked about the nooLite control core integrated into the Arduino Mega Server system. And it was also mentioned there that the core set of functions for controlling nooLite devices was implemented in the kernel and it was offered to everyone who wanted, by analogy, to add other necessary functions to the kernel. Now we will facilitate this task and add functions for wireless control of LED strips to the kernel.
First of all, we add the “main” function, which generates control commands for the nooLite MT1132 module .
void nooSendCommandLed(byte channel, byte command, byte r, byte g, byte b, byte format) {
byte buf[12];
ledLastState[0] = r;
ledLastState[1] = g;
ledLastState[2] = b;
for (byte i = 0; i < 12; i++) {
buf[i] = 0;
}
buf[0] = 85;
buf[1] = B01010000;
buf[2] = command;
buf[3] = format;
buf[5] = channel;
buf[6] = r;
buf[7] = g;
buf[8] = b;
int checkSum = 0;
for (byte i = 0; i < 10; i++) {
checkSum += buf[i];
}
buf[10] = lowByte(checkSum);
buf[11] = 170;
for (byte i = 0; i < (12); i++) {
Serial1.write(buf[i]);
}
}
This is the sister of the nooSendCommand function, which we covered in the first article. It is only slightly modified for the specific needs of controlling LED strips. Added installation of 6, 7, 8 bytes of the control command, which contain brightness on the three channels R, G, B. And an array is added
byte ledLastState[] = {127, 127, 127};
containing the last set color value of the LED strip. This is necessary so that you can turn the light on and off, while maintaining the set color value. If this is not done, then after turning it off and then turning it on, the color will reset to white, which is not very convenient.
And for working with LED strip, select the third channel
byte const NOO_CHANNEL_3 = 2;
You could choose any of the 32 channels that the nooLite MT1132 module works with.
Now we have the basic function that controls the operation of the nooLite SD111-180 LED strip controller, and, accordingly, the LED strip itself. And now we can start writing wrapper functions that will make it easier for us to write code to control the color of the glow of the tape.
Base wrap
void nooLed (byte ch, byte r, byte g, byte b) {
nooSendCommandLed(ch, 6, r, g, b, 3);
}
Just indicate the channel and the colors of the glow. At our disposal 16 million shades. Next, we write wrapper functions for the base colors (you can add such functions for your favorite colors).
void nooRed (byte ch, byte v) {nooSendCommandLed(ch, 6, v, 0, 0, 3);}
void nooGreen (byte ch, byte v) {nooSendCommandLed(ch, 6, 0, v, 0, 3);}
void nooBlue (byte ch, byte v) {nooSendCommandLed(ch, 6, 0, 0, v, 3);}
void nooYellow (byte ch, byte v) {nooSendCommandLed(ch, 6, v, v, 0, 3);}
void nooMagenta(byte ch, byte v) {nooSendCommandLed(ch, 6, v, 0, v, 3);}
void nooCyan (byte ch, byte v) {nooSendCommandLed(ch, 6, 0, v, v, 3);}
Just indicate the channel and the intensity of the glow in the range from 0 to 255. And a few more "conceptual" functions. White, black and gray.
void nooBlack (byte ch) {nooSendCommandLed(ch, 6, 0, 0, 0, 3);}
void nooWhite (byte ch) {nooSendCommandLed(ch, 6, 255, 255, 255, 3);}
void nooGray (byte ch, byte v) {nooSendCommandLed(ch, 6, v, v, v, 3);}
And the last function that we will add to the nooLite core in the Arduino Mega Server system is the function to restore the last color of the glow of the tape (the nooLite modules themselves do not remember this value). This function is used to turn on the tape (after turning it off, which resets the color to white).
void nooSetLedLastState(byte ch) {
nooSendCommandLed(ch, 6, ledLastState[0], ledLastState[1], ledLastState[2], 3);
}
Here is the complete code for the new nooLite management kernel.
Full kernel code
/*
Modul nooLite for Due
part of Arduino Mega Server project
*/
#ifdef NOO_FEATURE
byte const PIN_TX = 18; // TX PIN (to RX noolite)
byte const PIN_RX = 19; // RX PIN (to TX noolite)
byte const NOO_CHANNEL_1 = 0; // channel (address) 0...31 (MT1132)
byte const NOO_CHANNEL_2 = 1;
byte const NOO_CHANNEL_3 = 2;
byte const NOO_CHANNEL_4 = 3;
byte const NOO_CHANNEL_5 = 4;
void nooInit() {
Serial1.begin(9600);
modulNoo = MODUL_ENABLE;
started("nooLite");
}
void nooWork() {
}
void nooSendCommand(byte channel, byte command, byte data, byte format) {
byte buf[12];
for (byte i = 0; i < 12; i++) {
buf[i] = 0;
}
buf[0] = 85;
buf[1] = B01010000;
buf[2] = command;
buf[3] = format;
buf[5] = channel;
buf[6] = data;
int checkSum = 0;
for (byte i = 0; i < 10; i++) {
checkSum += buf[i];
}
buf[10] = lowByte(checkSum);
buf[11] = 170;
for (byte i = 0; i < (12); i++) {
Serial1.write(buf[i]);
}
/*
Serial.println(">");
for (byte i = 0; i < (12); i++) {
Serial.print(buf[i]);
}
Serial.println();
*/
}
byte ledLastState[] = {127, 127, 127};
void nooSendCommandLed(byte channel, byte command, byte r, byte g, byte b, byte format) {
byte buf[12];
ledLastState[0] = r;
ledLastState[1] = g;
ledLastState[2] = b;
for (byte i = 0; i < 12; i++) {
buf[i] = 0;
}
buf[0] = 85;
buf[1] = B01010000;
buf[2] = command;
buf[3] = format;
buf[5] = channel;
buf[6] = r;
buf[7] = g;
buf[8] = b;
int checkSum = 0;
for (byte i = 0; i < 10; i++) {
checkSum += buf[i];
}
buf[10] = lowByte(checkSum);
buf[11] = 170;
for (byte i = 0; i < (12); i++) {
Serial1.write(buf[i]);
}
}
// command data format
void nooBind (byte ch) {nooSendCommand(ch, 15, 0, 0);}
void nooUnbind (byte ch) {nooSendCommand(ch, 9, 0, 0);}
void nooOn (byte ch) {nooSendCommand(ch, 2, 0, 0);}
void nooOff (byte ch) {nooSendCommand(ch, 0, 0, 0);}
void nooTrigger(byte ch) {nooSendCommand(ch, 4, 0, 0);}
void nooCancel (byte ch) {nooSendCommand(ch, 10, 0, 0);}
void nooUp (byte ch) {nooSendCommand(ch, 3, 0, 0);}
void nooDown (byte ch) {nooSendCommand(ch, 1, 0, 0);}
void nooRevers (byte ch) {nooSendCommand(ch, 5, 0, 0);}
void nooValue (byte ch, byte v) {nooSendCommand(ch, 6, v, 1);}
void nooLed (byte ch, byte r, byte g, byte b) {nooSendCommandLed(ch, 6, r, g, b, 3);}
void nooBlack (byte ch) {nooSendCommandLed(ch, 6, 0, 0, 0, 3);}
void nooWhite (byte ch) {nooSendCommandLed(ch, 6, 255, 255, 255, 3);}
void nooGray (byte ch, byte v) {nooSendCommandLed(ch, 6, v, v, v, 3);}
void nooRed (byte ch, byte v) {nooSendCommandLed(ch, 6, v, 0, 0, 3);}
void nooGreen (byte ch, byte v) {nooSendCommandLed(ch, 6, 0, v, 0, 3);}
void nooBlue (byte ch, byte v) {nooSendCommandLed(ch, 6, 0, 0, v, 3);}
void nooYellow (byte ch, byte v) {nooSendCommandLed(ch, 6, v, v, 0, 3);}
void nooMagenta(byte ch, byte v) {nooSendCommandLed(ch, 6, v, 0, v, 3);}
void nooCyan (byte ch, byte v) {nooSendCommandLed(ch, 6, 0, v, v, 3);}
void nooSetLedLastState(byte ch) {nooSendCommandLed(ch, 6, ledLastState[0], ledLastState[1], ledLastState[2], 3);}
#endif // NOO_FEATURE
That's all, with this, with the description of the kernel add-ons, we end and go to the AMS LED strip color management interface.
LED Strip Management Interface
In previous articles, you remember the nooLite instrument control interface of the Arduino Mega Server system. We will not be particularly sophisticated and just add another section to the existing three (two sections for controlling devices and one section for “binding” / “untying” the modules).

Screenshots of other sections with detailed explanations can be seen in previous articles on the integration of AMS with the nooLite system. The new section makes it possible to turn on and off the LED strip and select a glow color from several predefined options.
This is just an example of integration and you can make any other interface based on this example. You can make color choices, smoothly change colors, or provide scenarios for turning on, off, and changing the color of the backlight, etc., etc.
Domestic kitchen
The control interface is only part of the features that the Arduino Mega Server provides with the integrated nooLite instrument control core. And this, so to speak, is the surface part of the iceberg. And the underwater part is the ability to use wrapper functions in the code of the system itself. You can easily, literally in one line of code, control the glow of the tape and use it to indicate any internal system parameters (temperature, security functions, turning on devices, etc.) and external ones that come via the network in the form of commands from other devices of the Smart Home .
And here we go to the most interesting.
Magic crystal
Now let's talk actually about the "magic crystal." What is it about? Imagine any artificial environment of a person - the interior of an apartment or office, a hall for holding any events, the urban environment of our cities (stops, poles, curbstones, etc.), etc. So, the idea is that at the current level of technology development, any subject can be made an “indicator” of any events and parameters. You can go even further and make any subject interactive or “lively”, you can also equip any subject with a distinctive voice, etc. (hi, Alice!), But this is the topic of another article, we’ll talk about this another time.
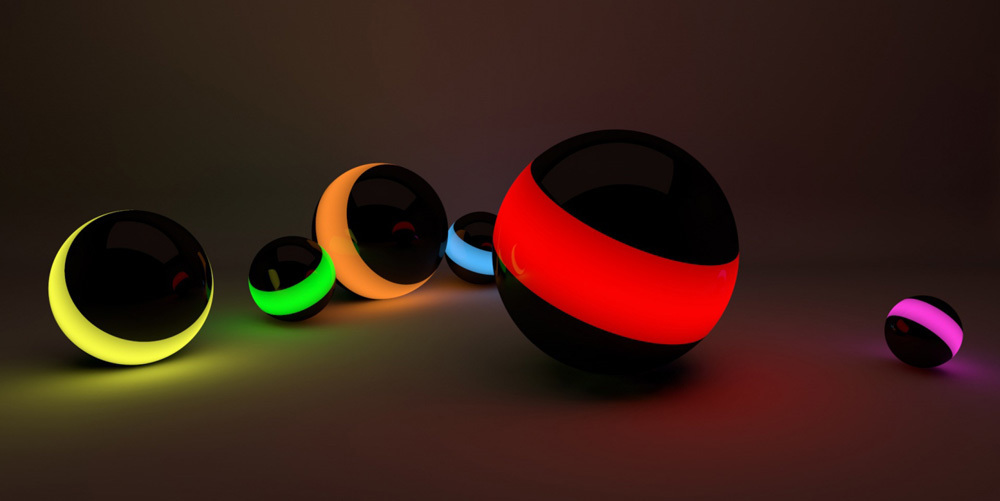
Take, for example, your room. On the shelf, next to the TV, or just in the middle of “creative mess” on the table, there may be a small object, for example, a “magic ball” which, depending on some parameters or events, changes its color, shade or brightness of the glow. It does not bother anyone and does not attract attention, it does not make any noise, but you just need to take a quick look at it and it becomes clear to you what temperature is on the street, whether you received an email by email, how much beer is left in the refrigerator, is it time to feed the fish or take sclerosis pills prescribed by your doctor.

Particularly important events “magic crystal” can be indicated by blinking or even sound. And you set all the parameters of this system yourself, depending on your needs and your imagination: the color and intensity of the glow, the events and parameters that the “magic crystal” reacts to, etc. There can be several such “crystals” and they can be any kind, not necessarily in the form of a ball.
Now from small and “invisible” things, let's move on to medium forms. It can be floor or ceiling lighting, luminous walls (parts of walls) or niches, etc. interior elements. For example, a backlight can serve as a color clock, when at night it changes light depending on time, and in the morning it gradually becomes brighter and serves as a “natural” alarm clock.

And finally, this is an inexhaustible storehouse of ideas for various performances and design of mass events, such as parties, corporate events, etc. meeting people, where a small magic crystal turns into 2-meter cubes and balls that change color depending on , for example, the results of voting for or against something.
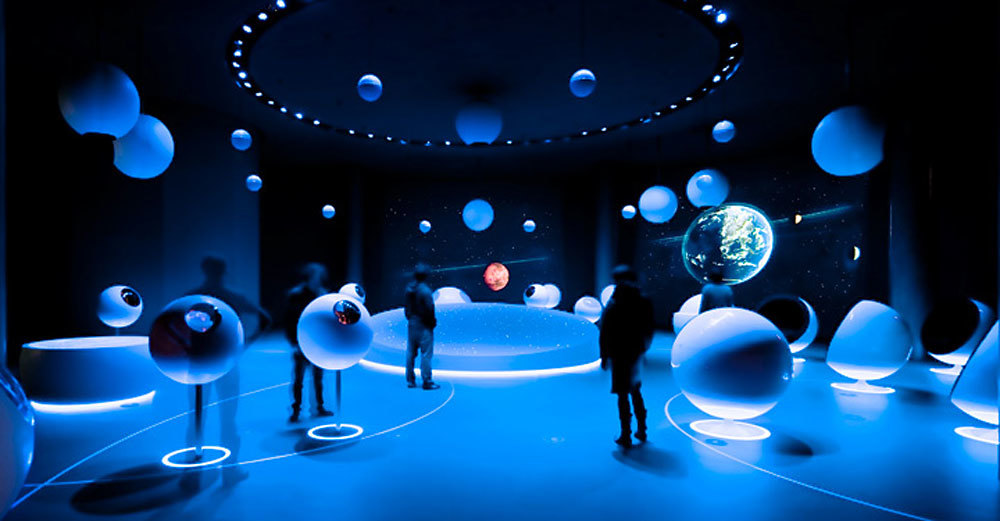
Conclusion
And all you need to realize all these ideas is the Arduino Mega Server , the nooLite SD111-180 wireless LED strip controller and a little bit of imagination. All the innovations described in this article will appear in the next 0.15 version of the AMS system, and the most impatient of you can take the nooLite kernel module code from this article and start working wonders right now.
Previous articles:
nooLite Wireless Equipment and Smart Home (Part 1). Arduino
nooLite Wireless Equipment and Smart Home (Part 2). Arduino Mega Server
nooLite Wireless Equipment and Smart Home (Part 3).
Arduino Mega Server soldering station promo on Youtube demonstrating how to work with a real
Arduino Mega Server promo system