JavaScript Guide, Part 2: Code Style and Program Structure
- Transfer
- Tutorial
Today we publish the translation of the next part of the javascript guide. Here we will talk about the style of the code and the lexical structure of the programs.
→ Part 1: first program, language features, standards
→ Part 2: code style and program structure
→ Part 3: variables, data types, expressions, objects
→ Part 4: functions
→ Part 5: arrays and cycles
→ Part 6: exceptions, semicolon, template literals
→ Part 7: strict mode, this keyword, events, modules, mathematical calculations
→ Part 8: an overview of the capabilities of the ES6 standard
→ Part 9: an overview of the capabilities of the ES7, ES8, and ES9 standards
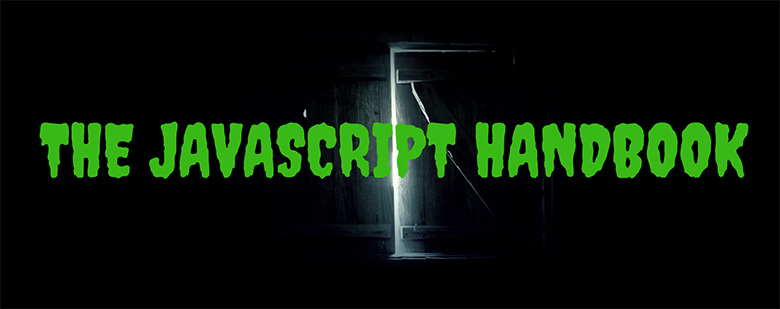
A “programming style,” or “coding standard,” or “code style,” is a set of conventions that are used when writing programs. They regulate the specifics of code design and the use of constructions that allow ambiguity. In our case, we are talking about programs written in JavaScript. If a programmer is working on a project himself, then the style of code used by him represents his “contract” with himself. In the case of a team, these are agreements that are used by all team members. Code written using a set of rules makes the code base of a software project uniform, improves readability and clarity of the code.
There are quite a few style guides. Here are 2 of them that are used in the JavaScript world most often:
You can easily choose any of them or come up with your own rules. The most important thing is to consistently use the same rules when working on a certain project. In this case, if, for example, you adhere to one set of rules, and in an existing project, on which you need to work, your own rules are used, you need to follow the rules of the project.
Code formatting can be done manually, or you can use automation tools for this process. In fact, formatting a JS code and checking it before launch is a separate large topic. Here is one of our publications on the relevant tools and features of their use.
The author of this material, as an example of his own style guide, cites a set of rules that he tries to follow when drawing up the code. He says that in the code of examples he is guided by the latest version of the standard available in modern versions of browsers. This means that in order to run such code in legacy browsers, you will need to use a transpiler, such as Babel . JS-transpilers allow you to translate code written using new language features, so that it can be executed in browsers that do not support these new features. The transpiler can provide support for language features that are not yet standard, that is, not implemented even in the most advanced browsers.
Here is a list of the rules in question.
Here is an example of a pair of simple switch functions:
if . Here are some ways to write a conditional statement
for . For the organization of cycles is used either a standard construction
while . Here is a schematic example of a loop
do . Here is the loop structure
switch . Below is a conditional statement
try . Here are some design options
Let's talk about the building blocks of javascript code. In particular, the use of Unicode encoding, semicolons, spaces, case sensitivity of a language, comments, literals, identifiers, and reserved words.
JavaScript code is presented using Unicode encoding. This, in particular, means that in the code, as variable names, you can use, say, emoticon characters. To do so, of course, is not recommended. It is important here that the names of identifiers, taking into account some rules , can be written in any language, for example, in Japanese or in Chinese.
The syntax of JavaScript is similar to the syntax of C. You can meet many projects in which, at the end of each line, there is a semicolon. However, semicolons at the end of lines in JavaScript are optional. In most cases, you can do without a semicolon. Developers who, prior to JS, used languages in which the semicolon is not used, tend to avoid them in JavaScript.
If you, while writing code, do not use strange constructions, or do not start a line with a parenthesis or a square bracket, then you will not make a mistake in 99.9% of cases (if anything - a linter can warn you about a possible error). The “strange constructions”, for example, include the following:
Whether to use a semicolon or not is a personal matter for each programmer. The author of this guide, for example, says that he decided not to use semicolons where they are not needed; as a result, they are extremely rare in the examples given here.
JavaScript does not pay attention to spaces. Of course, in certain situations the absence of a space will lead to an error (as well as an inappropriate space where it should not be), but very often there is no difference between the absence of a space in a certain place of the program and the presence of one or several spaces. A similar statement holds true not only for spaces, but also for newlines, and for tabs. This is especially noticeable, for example, on minifitsirovanny code. Take a look, for example, what the code processed with the Closure Compiler turns into .
In general, it should be noted that when formatting the program code, it is better not to go to extremes, adhering to some set of rules.
JavaScript is a case-sensitive language. This means that it distinguishes, for example, the names of variables
You can use two types of comments in JavaScript. The first type is single-line comments:
They, as the name implies, are located in one line. A comment is everything that follows characters
The second type is multi-line comments:
Here the comment is everything that is between the combination of characters
A literal is a certain value written in the source code of a program. For example, it can be a string, a number, a boolean value, or a more complex structure — an object literal (allows you to create objects, is framed by curly brackets), or an array literal (allows you to create arrays, is framed with square brackets). Here are some examples:
There will be no special benefit from launching a program in which such constructions are found. In order to work with literals in programs, they are first assigned to variables or passed to functions.
An identifier is a sequence of characters that can be used to identify a variable, function, object. It can start with a letter, a dollar sign (
The dollar sign is typically used when creating identifiers that store references to DOM elements.
Below is a list of words that are reserved by language. You cannot use them as identifiers.
Today we discussed the style and structure of programs written in JavaScript. Next time we'll talk about variables, data types, expressions, and objects.
Dear readers! What javascript style guide do you use?

→ Part 1: first program, language features, standards
→ Part 2: code style and program structure
→ Part 3: variables, data types, expressions, objects
→ Part 4: functions
→ Part 5: arrays and cycles
→ Part 6: exceptions, semicolon, template literals
→ Part 7: strict mode, this keyword, events, modules, mathematical calculations
→ Part 8: an overview of the capabilities of the ES6 standard
→ Part 9: an overview of the capabilities of the ES7, ES8, and ES9 standards
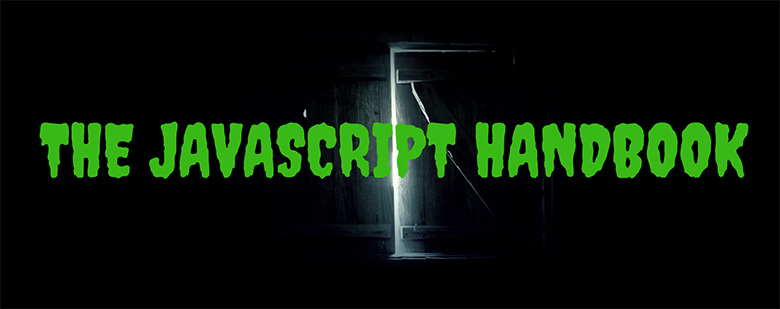
Programming style
A “programming style,” or “coding standard,” or “code style,” is a set of conventions that are used when writing programs. They regulate the specifics of code design and the use of constructions that allow ambiguity. In our case, we are talking about programs written in JavaScript. If a programmer is working on a project himself, then the style of code used by him represents his “contract” with himself. In the case of a team, these are agreements that are used by all team members. Code written using a set of rules makes the code base of a software project uniform, improves readability and clarity of the code.
There are quite a few style guides. Here are 2 of them that are used in the JavaScript world most often:
You can easily choose any of them or come up with your own rules. The most important thing is to consistently use the same rules when working on a certain project. In this case, if, for example, you adhere to one set of rules, and in an existing project, on which you need to work, your own rules are used, you need to follow the rules of the project.
Code formatting can be done manually, or you can use automation tools for this process. In fact, formatting a JS code and checking it before launch is a separate large topic. Here is one of our publications on the relevant tools and features of their use.
The style used in this guide
The author of this material, as an example of his own style guide, cites a set of rules that he tries to follow when drawing up the code. He says that in the code of examples he is guided by the latest version of the standard available in modern versions of browsers. This means that in order to run such code in legacy browsers, you will need to use a transpiler, such as Babel . JS-transpilers allow you to translate code written using new language features, so that it can be executed in browsers that do not support these new features. The transpiler can provide support for language features that are not yet standard, that is, not implemented even in the most advanced browsers.
Here is a list of the rules in question.
- Alignment To align the code blocks, spaces are used (2 spaces at 1 level of alignment), tabs are not used.
- Semicolon. Semicolon is not used.
- Line length 80 characters (if possible).
- Single line comments. Such comments are used in the code.
- Multiline comments. These comments are used to document the code.
- Unused code. Unused code does not remain in the program in commented out form in case you need it later. Such code, if you still need it, can be found in the version control system, if it is used, or in some kind of programmer’s notes intended for storing such code.
- Commenting rules. There is no need to comment on obvious things, add comments to the code that do not help to understand its essence. If the code explains itself due to well-chosen function and variable names and JSDoc function descriptions, you should not add additional comments to this code.
- Variable declaration Variables are always declared explicitly to prevent contamination of a global object. The keyword is
var
not used. If the value of a variable is not planned to be changed during the execution of the program, it is declared as a constant (such constants are often also called “variables”) using a keywordconst
, using it by default - except when it is planned to change the value of a variable. In such cases, a keyword is usedlet
. - Constants. If some values in the program are constants, their names are made up of capital letters. For example -
CAPS
. To separate parts of names consisting of several words, the underscore (_
) is used. - Functions. To declare functions, use the arrow syntax. Regular function declarations apply only in special cases. In particular, in methods of objects or in designers. This is done because of the characteristics of the keyword
this
. Functions must be declared using a keywordconst
, and, if possible, the results of their work must be explicitly returned from them. It is not forbidden to use nested functions in order to hide certain auxiliary mechanisms from the main code.
Here is an example of a pair of simple switch functions:
const test = (a, b) => a + b
const another = a => a + 2
- Entity naming. The names of functions, variables and methods of objects always begin with a lowercase letter, names consisting of several words are written using camel-style (such names appear as
camelCase
). Only the names of constructor functions and classes begin with a capital letter. If you use a certain framework that imposes special requirements on the naming of entities, use the rules prescribed by it. File names must be in lowercase letters, individual words in the names are separated by a dash (-
). - Rules for constructing and formatting expressions.
if . Here are some ways to write a conditional statement
if
:if (condition) {
statements
}
if (condition) {
statements
} else {
statements
}
if (condition) {
statements
} else if (condition) {
statements
} else {
statements
}
for . For the organization of cycles is used either a standard construction
for
, an example of which is given below, or a cycle for of
. Loops for in
should be avoided - except when used in conjunction with the design .hasOwnProperty()
. Here is the cycle diagram for
:for (initialization; condition; update) {
statements
}
while . Here is a schematic example of a loop
while
:while (condition) {
statements
}
do . Here is the loop structure
do
:do {
statements
} while (condition);
switch . Below is a conditional statement
switch
:switch (expression) {
case expression:
statements
default:
statements
}
try . Here are some design options
try-catch
. The first example shows this construction without a block finally
, the second with such a block.try {
statements
} catch (variable) {
statements
}
try {
statements
} catch (variable) {
statements
} finally {
statements
}
- Gaps . Spaces should be used wisely, that is, so that they contribute to improving the readability of the code. So, put spaces after the key words, followed by an open parenthesis, they frame the operators applied to two operands (
+
,-
,/
,*
,&&
and others). Spaces are used inside the loopfor
, after each semicolon, to separate the parts of the loop header from each other. Space is put after comma. - Empty lines . Blank lines are allocated to code blocks containing operations that are logically related to each other.
- Quotes . When working with strings, single quotes are used (
'
), not double quotes ("
). Double quotes are usually found in HTML attributes, so using single quotes helps to avoid problems when working with HTML strings. If you need to perform certain operations with strings, which imply, for example, their concatenation, you should use template literals that are written with back quotes (`
).
The lexical structure of JavaScript code
Let's talk about the building blocks of javascript code. In particular, the use of Unicode encoding, semicolons, spaces, case sensitivity of a language, comments, literals, identifiers, and reserved words.
NicUnicode
JavaScript code is presented using Unicode encoding. This, in particular, means that in the code, as variable names, you can use, say, emoticon characters. To do so, of course, is not recommended. It is important here that the names of identifiers, taking into account some rules , can be written in any language, for example, in Japanese or in Chinese.
▍ semicolon
The syntax of JavaScript is similar to the syntax of C. You can meet many projects in which, at the end of each line, there is a semicolon. However, semicolons at the end of lines in JavaScript are optional. In most cases, you can do without a semicolon. Developers who, prior to JS, used languages in which the semicolon is not used, tend to avoid them in JavaScript.
If you, while writing code, do not use strange constructions, or do not start a line with a parenthesis or a square bracket, then you will not make a mistake in 99.9% of cases (if anything - a linter can warn you about a possible error). The “strange constructions”, for example, include the following:
return
variable
Whether to use a semicolon or not is a personal matter for each programmer. The author of this guide, for example, says that he decided not to use semicolons where they are not needed; as a result, they are extremely rare in the examples given here.
▍ Spaces
JavaScript does not pay attention to spaces. Of course, in certain situations the absence of a space will lead to an error (as well as an inappropriate space where it should not be), but very often there is no difference between the absence of a space in a certain place of the program and the presence of one or several spaces. A similar statement holds true not only for spaces, but also for newlines, and for tabs. This is especially noticeable, for example, on minifitsirovanny code. Take a look, for example, what the code processed with the Closure Compiler turns into .
In general, it should be noted that when formatting the program code, it is better not to go to extremes, adhering to some set of rules.
Реги Case Sensitivity
JavaScript is a case-sensitive language. This means that it distinguishes, for example, the names of variables
something
and Something
. The same goes for any ids.▍Comments
You can use two types of comments in JavaScript. The first type is single-line comments:
// Это комментарий
They, as the name implies, are located in one line. A comment is everything that follows characters
//
. The second type is multi-line comments:
/*
Многострочный
комментарий
*/
Here the comment is everything that is between the combination of characters
/*
and */
.ИтLiterals and identifiers
A literal is a certain value written in the source code of a program. For example, it can be a string, a number, a boolean value, or a more complex structure — an object literal (allows you to create objects, is framed by curly brackets), or an array literal (allows you to create arrays, is framed with square brackets). Here are some examples:
5
'Test'
true
['a', 'b']
{color: 'red', shape: 'Rectangle'}
There will be no special benefit from launching a program in which such constructions are found. In order to work with literals in programs, they are first assigned to variables or passed to functions.
An identifier is a sequence of characters that can be used to identify a variable, function, object. It can start with a letter, a dollar sign (
$
) or an underscore ( _
), it can contain numbers, and, if necessary, Unicode characters like emoticons (although, as already mentioned, it is better not to do so). Here are some examples of identifiers:Test
test
TEST
_test
Test1
$test
The dollar sign is typically used when creating identifiers that store references to DOM elements.
▍Reserved words
Below is a list of words that are reserved by language. You cannot use them as identifiers.
break
do
instanceof
typeof
case
else
new
var
catch
finally
return
void
continue
for
switch
while
debugger
function
this
with
default
if
throw
delete
in
try
class
enum
extends
super
const
export
import
implements
let
private
public
interface
package
protected
static
yield
Results
Today we discussed the style and structure of programs written in JavaScript. Next time we'll talk about variables, data types, expressions, and objects.
Dear readers! What javascript style guide do you use?
