Extending the functionality of EPLAN. Creating a simple Add-Ina in C #
Extending EPLAN functionality with Add-Ins in C #
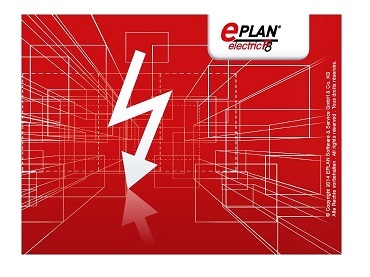
EPLAN Application
• Automotive industry
• Mechanical engineering
• Metallurgy
• Chemical and pharmaceutical industries
• Food industry
• Oil and gas production
• Pipeline transport
• Oil and gas processing
• Heat and power generation
• Electricity transmission and distribution
• Railway transport
• Water supply and sanitation
• Machine tool industry
• Light Industry
• Building Automation
Main platform modules
• EPLAN Electric P8 - A modular and scalable solution for electrical engineering, the automatic creation of design and working documentation.
• EPLAN Fluid - Software for the design of pneumatic / hydraulic automation, lubrication and cooling systems and the automatic creation of appropriate design and working documentation
• EPLAN ProPanel - 3D design of electrical cabinets with data transfer to production. Virtual three-dimensional modeling, the creation of two- and three-dimensional drawings, three-dimensional images of wire and route diagrams, the availability of templates for drilling equipment and integration with CNC machines
• EPLAN PrePlanning - software for preliminary (outline) design of objects and generation of project documentation.
• EPLAN Engineering Center - Solution for functional design. In this module, the user sets the boundary parameters of the project, and the design itself is carried out automatically by the system according to certain rules.
About the expansion of functionality
EPLAN is a flexible platform that allows you to expand functionality with scripts and Add-Ins. We will consider only Add-Ins, since scripts (scripts) give much less freedom of action.
Add-In is an add-on that complements and extends the basic functionality offered by EPLAN. Add-In is created using the EPLAN API, which, in turn, uses dotNET and supports 3 programming languages: Visual Basic, C ++ and C #. The procedure for creating Add-Ins is the same for all the above languages. EPLAN API information is available in the EPLAN API-Support manual (in * .chm format), which is supplied with the documentation.
The process of creating Add-Ina
Consider directly the process of creating Add-Ins.
1. For starters, since dotNET is used, we create a Class Library project in MS Visual Studio.
2. Next, we connect the EPLAN API library to the project.
3. We write the
Add-Ina initialization code To register and initialize Add-Ina, our class must inherit the IEplAddIn interface (for more details, see EPLAN API-Support). Create a menu item in the EPLAN Main Menu and add one Action to it.
using Eplan.EplApi.ApplicationFramework;
using Eplan.EplApi.Gui;
namespace Test
{
public class AddInModule:IEplAddIn
{
public bool OnRegister(ref bool bLoadOnStart)
{
bLoadOnStart = true;
return true;
}
public bool OnUnregister()
{
return true;
}
public bool OnInit()
{
return true;
}
public bool OnInitGui()
{
Menu OurMenu = new Menu();
OurMenu.AddMainMenu("Тест", Menu.MainMenuName.eMainMenuUtilities, "Информация по проекту", "ActionTest",
"Имя проекта, фирмы и дата создания", 1);
return true;
}
public bool OnExit()
{
return true;
}
}
}
The OnRegister and OnUnregister methods are called once, the first time you connect and remove Add-Ina, respectively.
The OnExit method is called when EPLANa is closed.
The OnInit method is called when EPLANa loads to initialize the Add-Ina.
The OnInitGui method is called when EPLANa loads to initialize the Add-Ina and user interface.
4. We write the action code (Action), which will be in our menu. The Action must inherit the IEplAction interface (more in EPLAN API-Support).
using System;
using System.Windows.Forms;
using Eplan.EplApi.ApplicationFramework;
using Eplan.EplApi.DataModel;
using Eplan.EplApi.HEServices;
namespace Test.Action_test
{
public class Action_Test:IEplAction
{
public bool OnRegister(ref string Name, ref int Ordinal)
{
Name = "ActionTest";
Ordinal = 20;
return true;
}
public bool Execute(ActionCallingContext oActionCallingContext)
{
SelectionSet Set = new SelectionSet();
Project CurrentProject = Set.GetCurrentProject(true);
string ProjectName = CurrentProject.ProjectName;
string ProjectCompanyName = CurrentProject.Properties.PROJ_COMPANYNAME;
DateTime ProjectCreationDate = CurrentProject.Properties.PROJ_CREATIONDATE;
MessageBox.Show("Название проекта: " + ProjectName + "\n" + "Название фирмы: " + ProjectCompanyName +
"\n" + "Дата создания проекта: " + ProjectCreationDate.ToShortDateString());
return true;
}
public void GetActionProperties(ref ActionProperties actionProperties)
{
}
}
}
The OnRegister method registers our Action with the specified name.
The Execute method is executed when an Actiona is called from the EPLAN platform. In this case, the Execute method selects the current project, reads three fields from it, namely, the project name, company name and creation date, and then displays them in a MessageBox .
The GetActionProperties method returns a description of our Actiona (for documentation only).
5. The name of the compiled DLL must comply with the following rule:
Eplan.EplAddIn.XXXX.dll
where XXXX is the name of your Add-Ina
Add-Ina Connection
Add-In is connected to the platform as follows:
1. Execute “Service Programs”
- “API-AddIns ...”
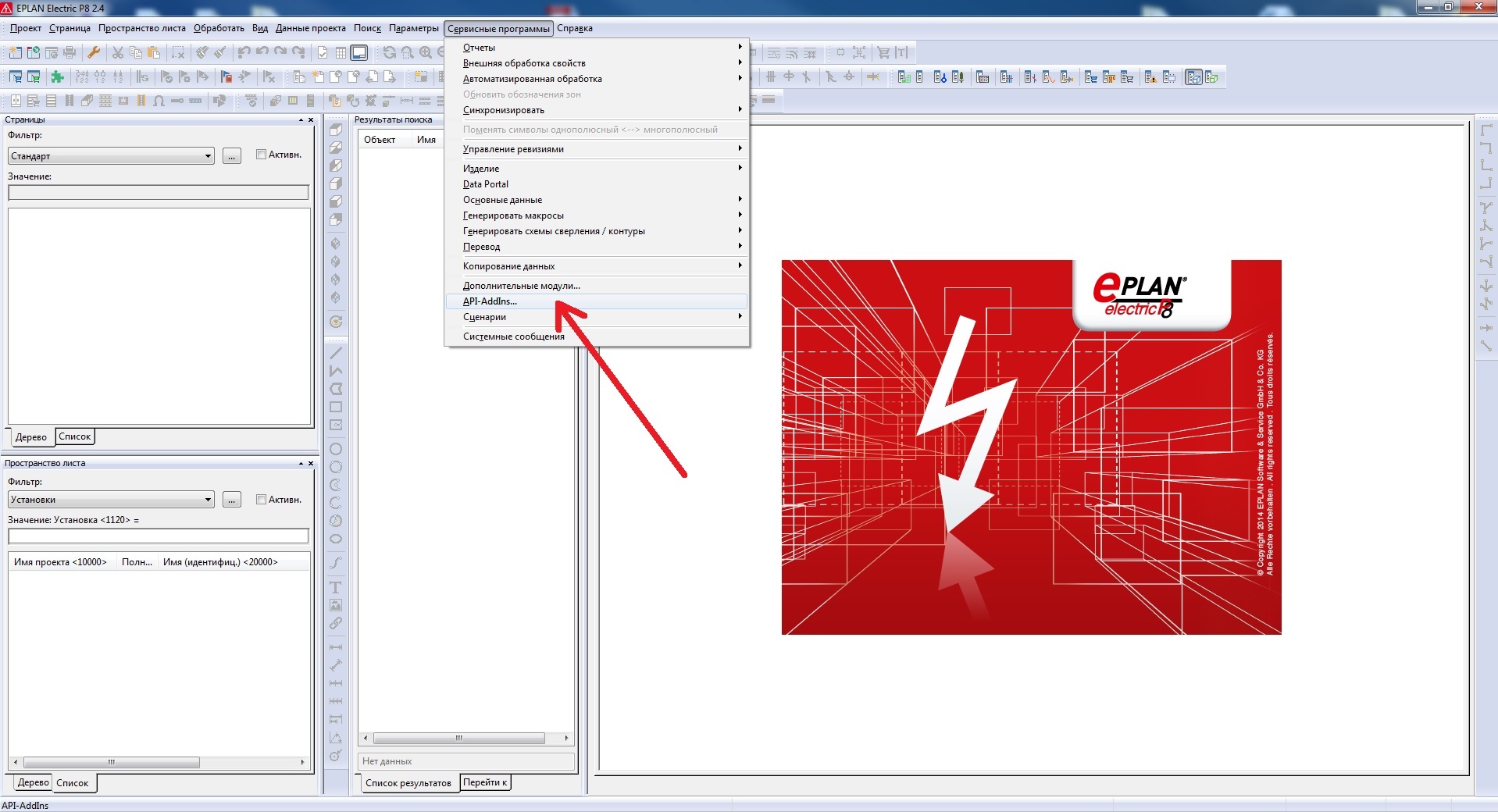
2. Click on the “Download” button
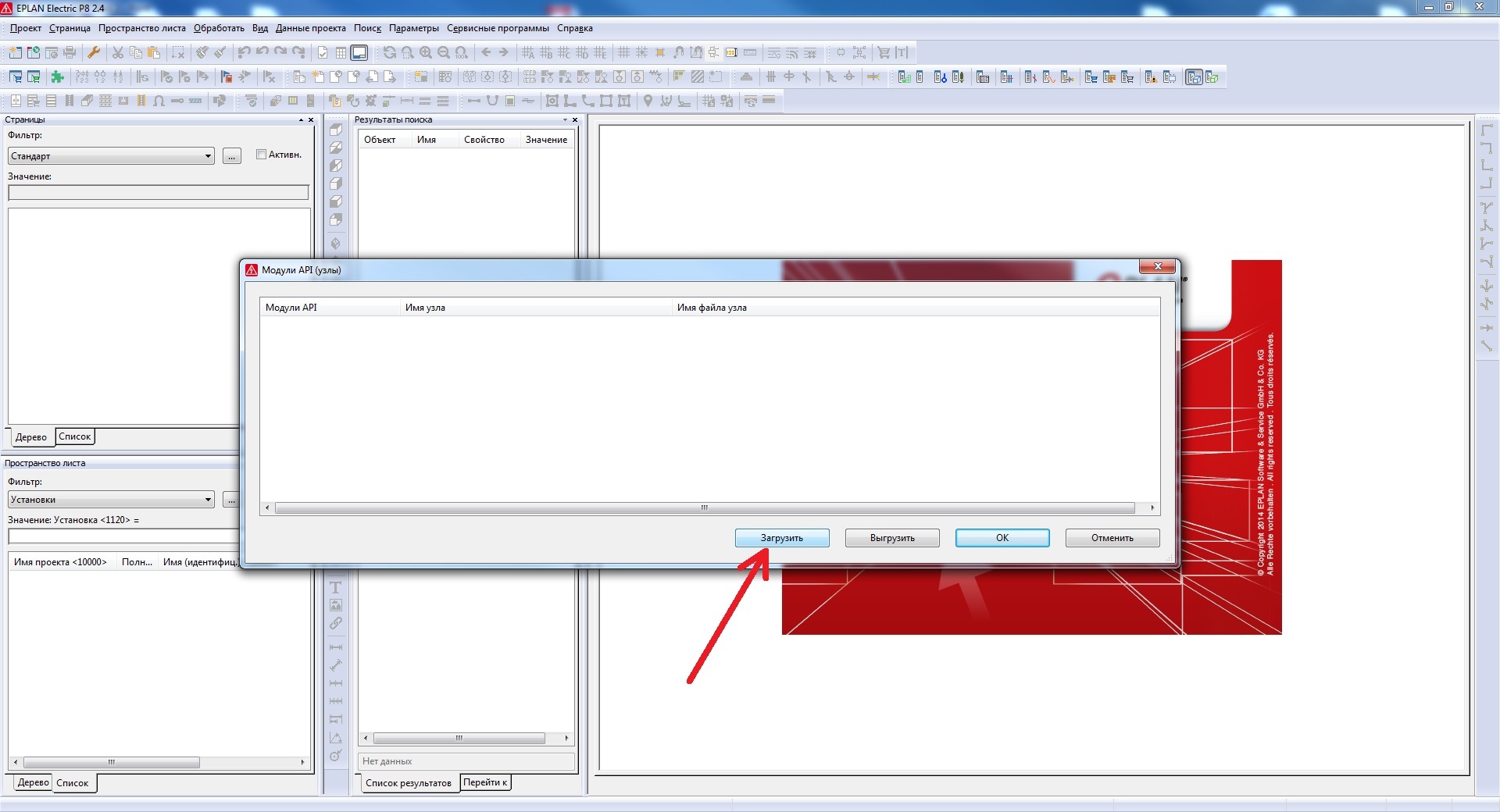
3. In the window that opens, select Add-In and click “Open”
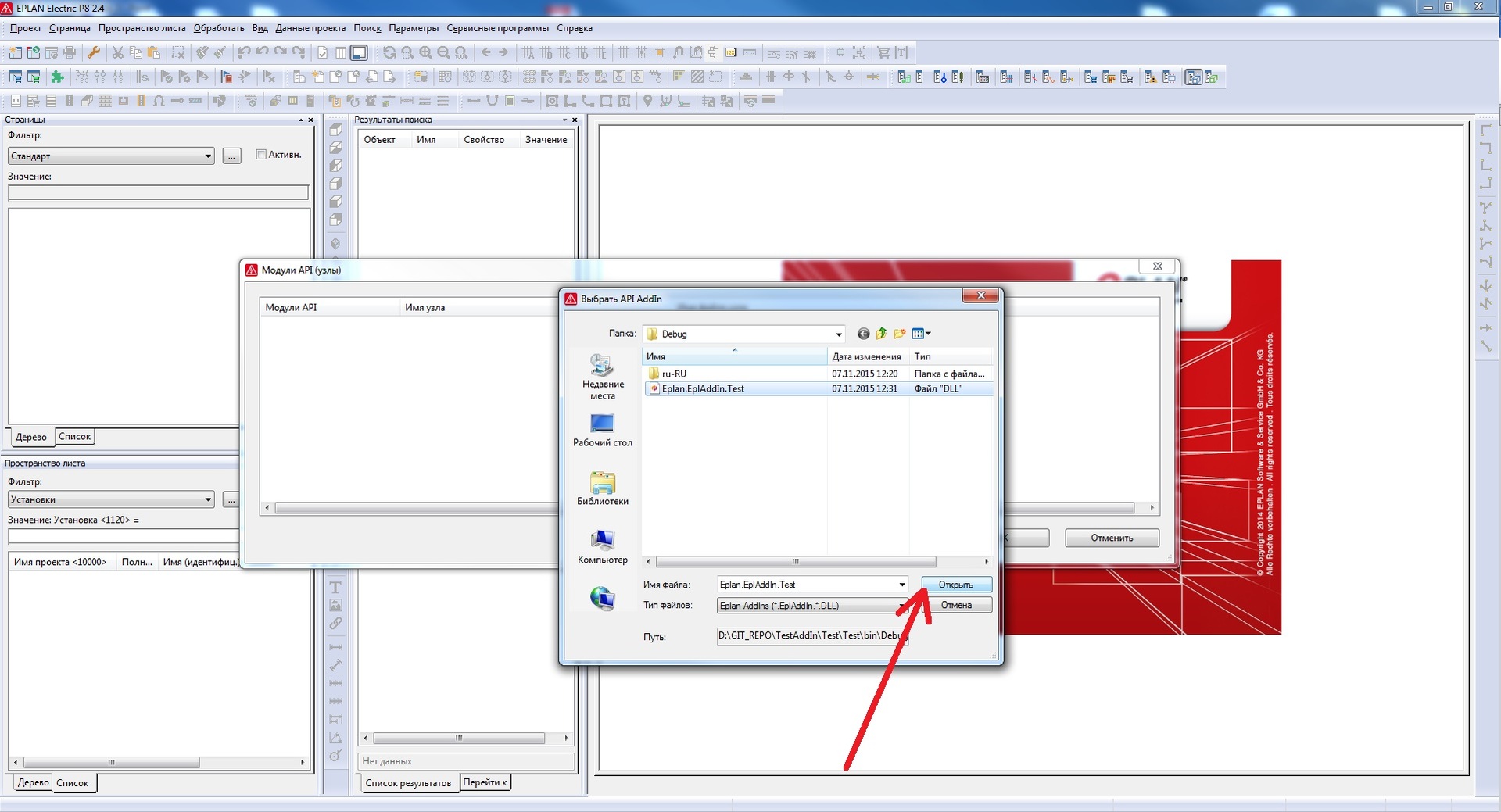
4. Click on the button "OK". The Add-In is up and ready to go.

When loading the Add-In, the “Test” menu item is initialized, opening which we see our action (Action) described in Action_Test.
Let's look at the result of Add-Ina:
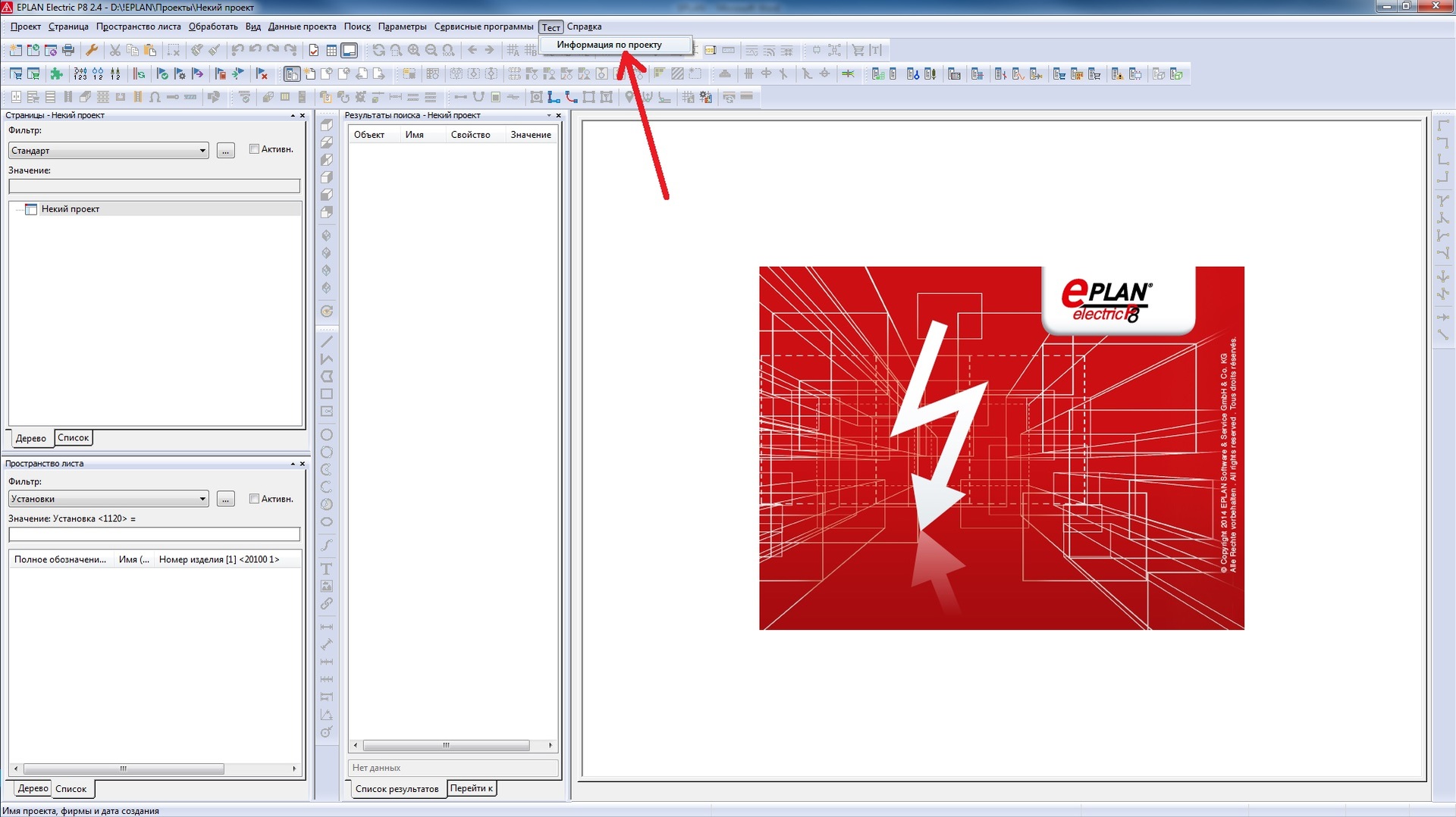
We click on the menu item "Test" - "Project Information".
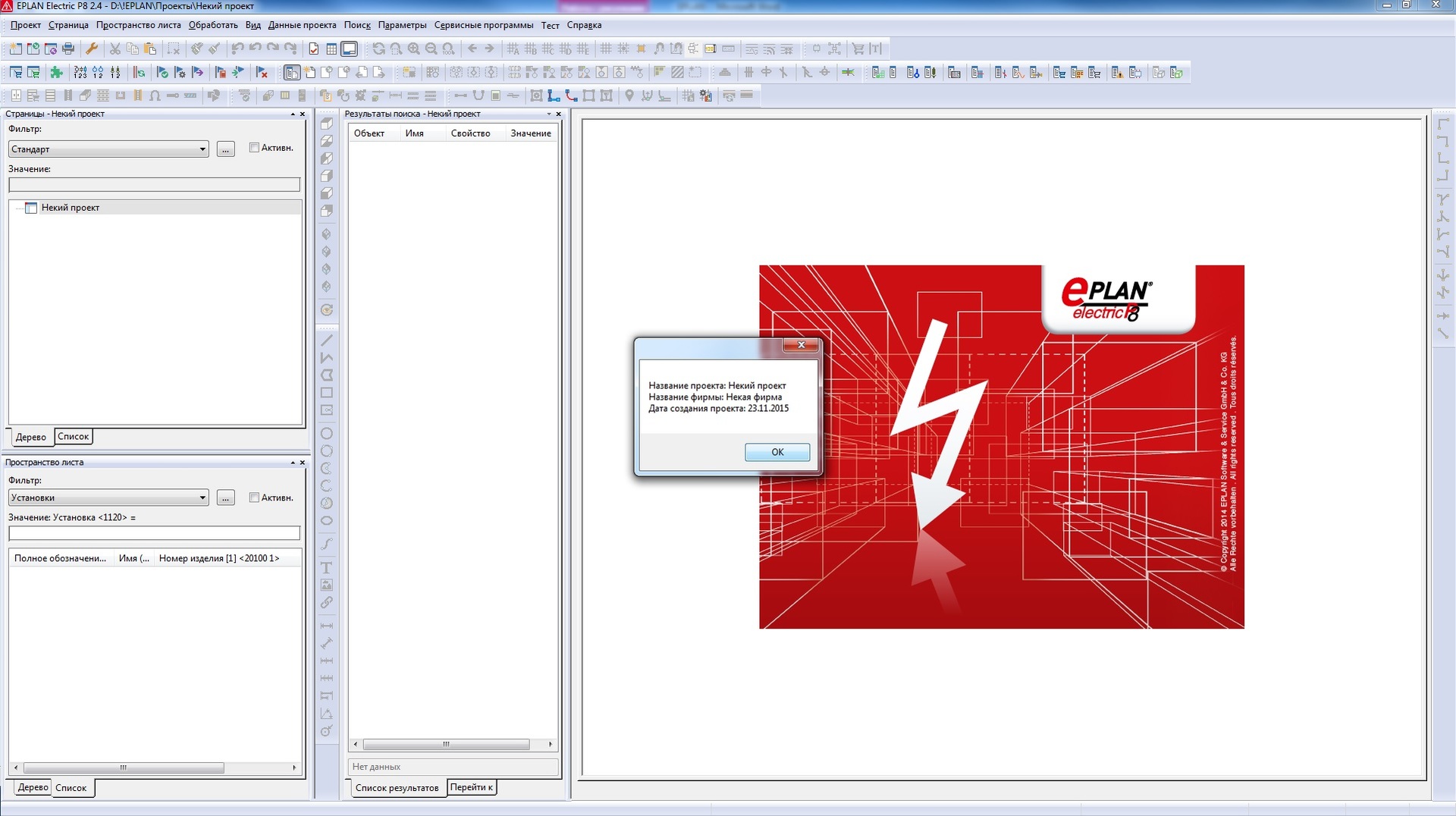
EPLAN platform provides great basic functionality. However, sometimes actions beyond its scope are required. In the simplest cases, you can create and connect scripts (scripts), but if you need deep access to the data, you need to use the API, create and use Add-ins.
useful links
1. EPLAN in Russia
2. ACS TP Forum