Working with the Mandrill Email Service API
- Tutorial
Mandrill is a powerful email service from MailChimp. It is one of the most convenient to use and configure from the family of the same type of service for sending email notifications. This service is convenient to use not only for sending certain letters of a commercial nature, but also for regular notifications from a personal site. Since emails sent from personal sites may be spammed, this will be another plus in favor of the decision to choose the services of this service.
For the Mandrill service to interact with the application, there is an API with a fairly wide range of capabilities, the main of which we have to get to know. In addition, there is the possibility of using a basic (free account) that allows you to send out up to 12,000 letters per month.
Since the implementation of interaction with the Mandrill API is supposed to be implemented in PHP, we take the library itself from here . You can use the installation via Composer or the standard installation (download, unpack and connect the necessary modules). If you chose the second installation option, then you need to find the “src” directory in the downloaded archive, it contains the files and directories of the necessary library.
In order to be able to use the API, you need to get an API key (meaning that you are already registered). We go into the account and click on the “Settings” item on the left in the menu and in the “API Keys” section we can see our key:
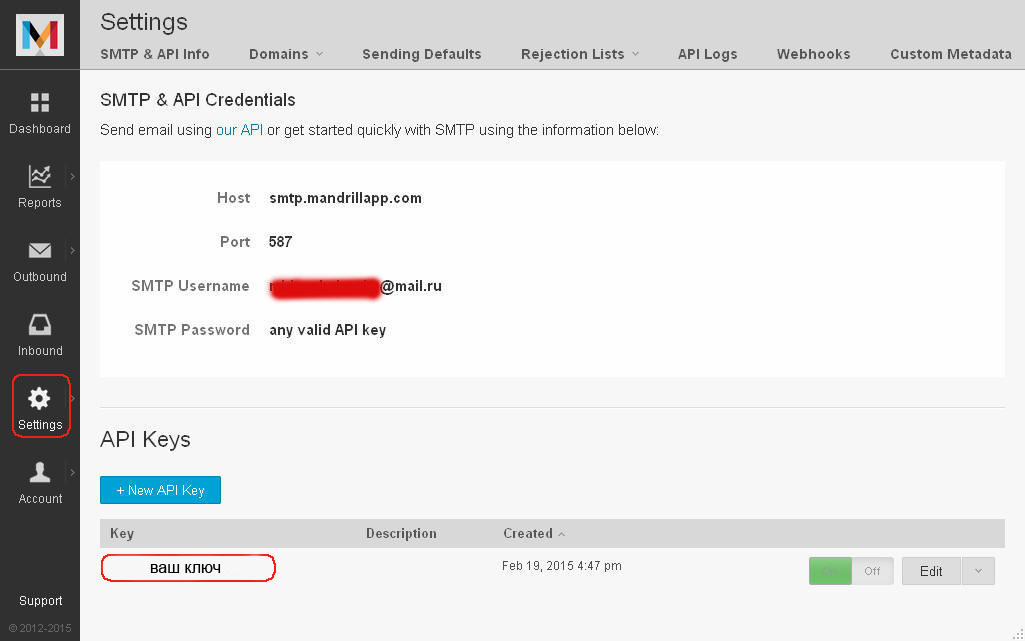
Next, you need to create a subaccount. To do this, again, in the left-hand menu, click on the “Outbound” item and after opening the corresponding settings section, click on the “Create a Subaccount” button:

To send a letter, you can use the pre-created template, which should be available in your account, or restrict yourself to sending a regular text message. It is also possible to format the letter in HTML format. To send an email, the following methods of the Mandrill_Messages class exist :
Table 1 . Description of the data structure fields for sending a letter
Table 2 . The structure of the array element used in the template_content parameter
The send () method allows you to send a text message, and the second method provides the ability to send letters using pre-created templates. Both methods return an array with the data structure presented in table. 3.
Table 3 . Response structure for each recipient
In order not to be distracted later, we will create a template immediately for the future. To do this, in the already familiar left-side menu, click on the “Outbound” item and after going to the appropriate section, click on the “Create a Template” button:
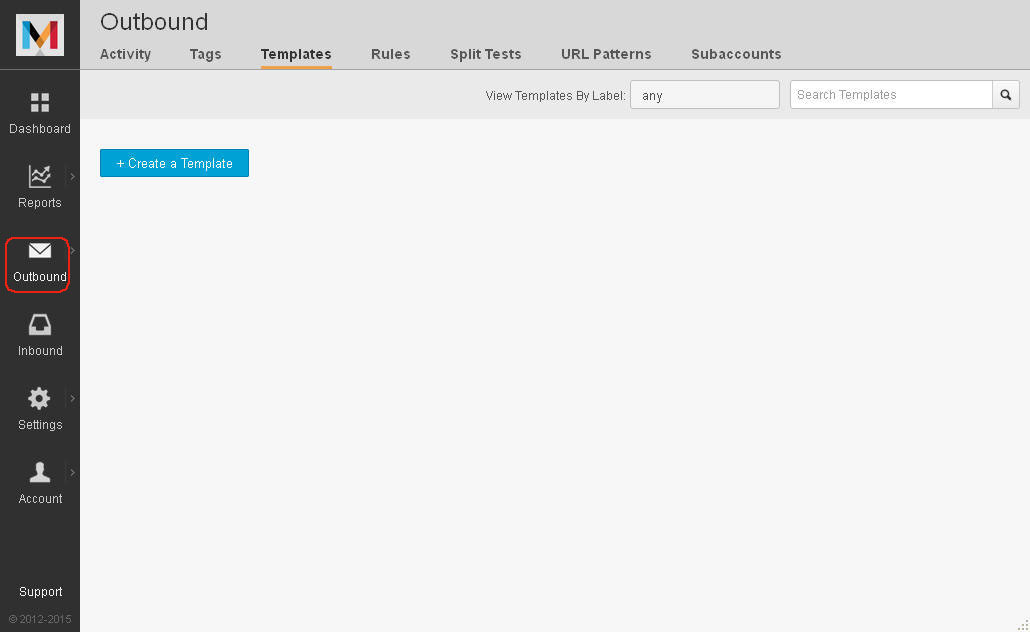
Here we will be asked to enter the name of the template. After entering one, click on the “Start Coding” button:

And already at the final stage, we need to fill out the proposed fields. Then in the right field you need to write the code for our future wildcard template. Please note that the template editor must be in “HTML” mode. After filling in all the fields we need, click the “Publish” button:

Now that everything is already set up, you can send letters. Source code for sending a letter:
The results of the two methods are presented below. The bottom image shows the result of applying the template.

We sent the same email using both methods available to us. In order to be able to see the differences. As an example, an image and a PDF document were attached to the letter.
Also, work with dynamic content and templates was demonstrated. The difference in using a template from dynamic content is that a label is specified for the template.
This label will be replaced in the future by its content. And for dynamic content, the following attribute is specified in the HTML tag.
where, section-name is a unique identifier, which means that the data of the specified identifier will be inserted into the HTML tag containing it. It is also worth considering that the template elements specified in the global_merge_vars field (see table 1 ) will be replaced by the corresponding elements specified in the vars field of the merge_vars structure for the specified email addresses in the rcpt field of the merge_vars structure "
Consider the following example:
Here the label will be redefined only for the recipient with the address “example_1@gmail.com”. Accordingly, the label in the template with the name “ NAME ” will be replaced with the name “ Alex ”, for the rest of the recipients the contents of the label will remain “ Lucas ”.
When using both methods to send letters, the following factors must be considered:
The list of possible errors is contained in the table. 4.
Table 4 . The list of possible errors when sending a letter is unsuccessful
For the Mandrill service to interact with the application, there is an API with a fairly wide range of capabilities, the main of which we have to get to know. In addition, there is the possibility of using a basic (free account) that allows you to send out up to 12,000 letters per month.
API usage
Since the implementation of interaction with the Mandrill API is supposed to be implemented in PHP, we take the library itself from here . You can use the installation via Composer or the standard installation (download, unpack and connect the necessary modules). If you chose the second installation option, then you need to find the “src” directory in the downloaded archive, it contains the files and directories of the necessary library.
In order to be able to use the API, you need to get an API key (meaning that you are already registered). We go into the account and click on the “Settings” item on the left in the menu and in the “API Keys” section we can see our key:
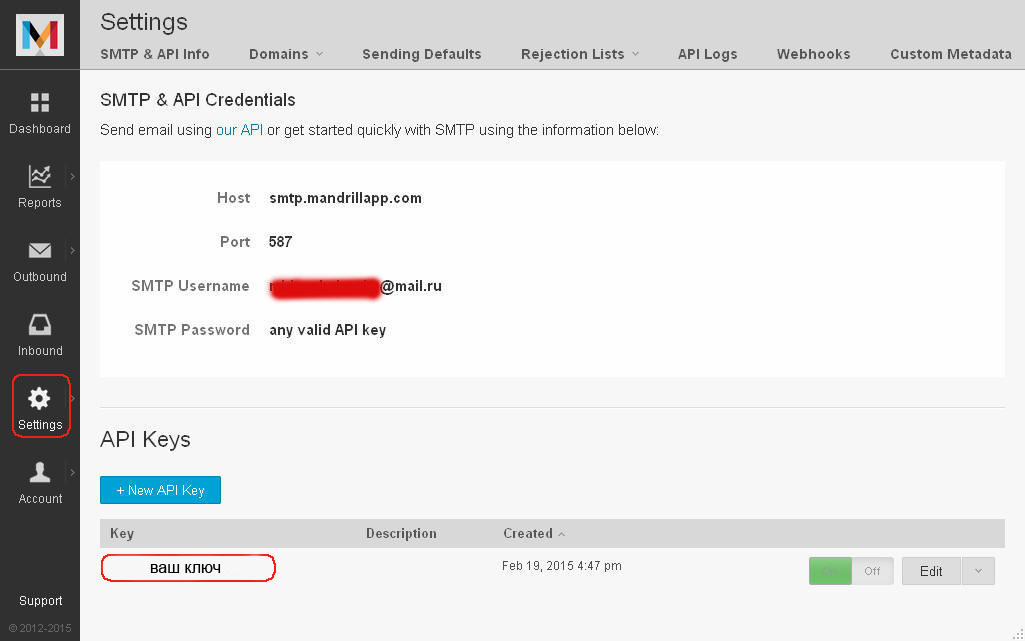
Next, you need to create a subaccount. To do this, again, in the left-hand menu, click on the “Outbound” item and after opening the corresponding settings section, click on the “Create a Subaccount” button:

To send a letter, you can use the pre-created template, which should be available in your account, or restrict yourself to sending a regular text message. It is also possible to format the letter in HTML format. To send an email, the following methods of the Mandrill_Messages class exist :
- array send (message [, async = false [, ip_pool = null [, send_at = null]]]) - as the parameter message, you need to pass the data structure filled in as an array, given in Table. 1 . Async parameter - sets the background batch message sending. As the ip_pool parameter, the name of the allocated pool of ip addresses is required, as can be seen from the description of the function prototype, this parameter is optional. And as the last send_at parameter, you can specify the time in UTC timestamp format YYYY-MM-DD HH: MM: SS for the scheduled sending of the letter. But this will require a paid account with a positive balance;
- array sendTemplate (template_name, template_content, message [, async = false [, ip_pool = null [, send_at = null]]]) - this method differs from the previous one by the presence of two additional parameters. The first parameter to template_name specifies the name of an existing template. And the second parameter template_content is an array of dynamic content elements, the data structure of which is described in the table. 2 . More details can be found here .
Table 1 . Description of the data structure fields for sending a letter
Parameter | Description |
html string | Text with HTML markup content. |
text string | Text of the letter. |
subject string | Letter subject. |
from_email string | Email of the sender. |
from_name string | Sender name. |
to array | An array of “to” elements containing sender information. |
array | An array of global_merge_vars elements containing global template label data. |
merge_vars array | An array of merge_vars elements containing template label data. |
subaccount string | The unique identifier for the subaccount. |
attachments array | An array of attachments added to the email. |
images array | An array of images added to the letter. |
Array parameter structure to | |
---|---|
email string | Email addressee. |
name string | The name of the recipient. |
type string | Type of title. The default is “to”. Possible options (to, cc, bcc). |
Array parameter structure global_merge_vars | |
name string | Variable name, case sensitive. The variable name cannot begin with the character “_”. |
content string | The value of the variable. |
Merge_vars array parameter structure | |
rcpt string | The email address of the recipient to which the local wildcard will be applied. |
vars array | An array of “vars” elements containing local template label data. The structure of the vars array parameter is identical to the structure of the global_merge_vars array parameter. |
Attributes Array Parameter Structure | |
type string | MIME type attachments. |
name string | File name. |
content string | The contents of the file must be base64 encoded. |
Parameter structure of the images array. | |
type string | MIME type of the file - must be an image. |
name string | File name. |
content string | The contents of the file must be base64 encoded. |
Table 2 . The structure of the array element used in the template_content parameter
Parameter | Description |
name string | The name of the label. |
content string | The content of the tag. |
The send () method allows you to send a text message, and the second method provides the ability to send letters using pre-created templates. Both methods return an array with the data structure presented in table. 3.
Table 3 . Response structure for each recipient
Parameter | Description |
email string | Email addressee. |
status string | Sending status: sent, queued, scheduled, rejected or sending is not possible. |
string | The reason for the rejection of the letter delivery. Maybe one of the following options: rejected, hard-bounce, soft-bounce, spam, unsub, custom, invalid-sender, invalid, test-mode-limit, rule |
_id string | The unique identifier of the sent message. |
In order not to be distracted later, we will create a template immediately for the future. To do this, in the already familiar left-side menu, click on the “Outbound” item and after going to the appropriate section, click on the “Create a Template” button:
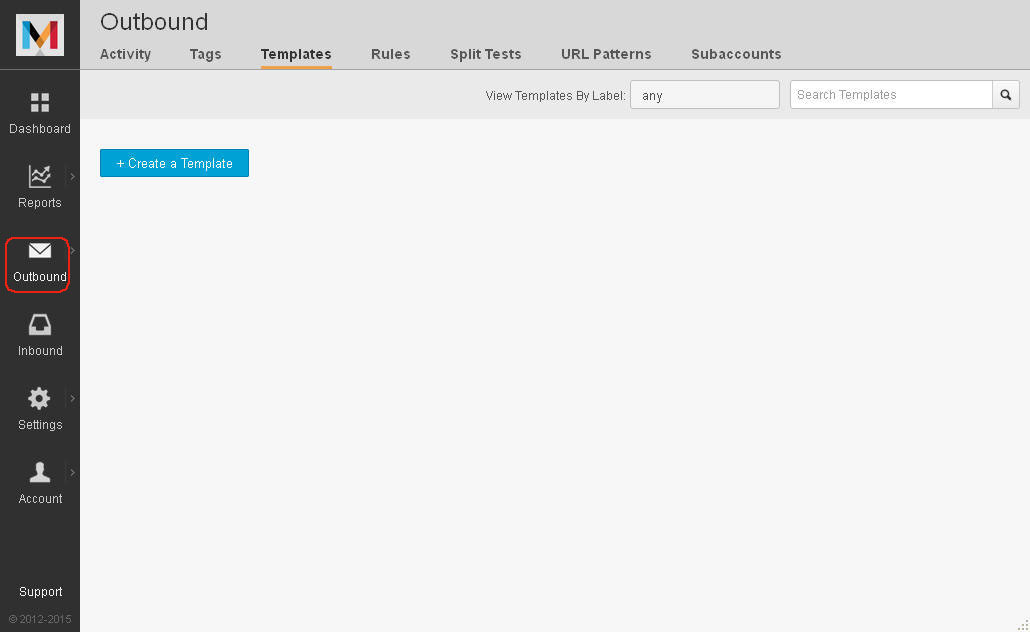
Here we will be asked to enter the name of the template. After entering one, click on the “Start Coding” button:

And already at the final stage, we need to fill out the proposed fields. Then in the right field you need to write the code for our future wildcard template. Please note that the template editor must be in “HTML” mode. After filling in all the fields we need, click the “Publish” button:

Now that everything is already set up, you can send letters. Source code for sending a letter:
// Подключение библиотеки Mandrill
require_once ('Mandrill.php');
$mail = new Mandrill('API key');
/* Блок динамического контента */
$tpl_con = array(
array(
'name' => 'my-label',
'content' => 'Моя рассылка
'
)
);
$data = array(
'html' => 'It is html text',
'text' => 'It is simple text',
'subject' => 'Hi friend',
'from_email' => 'friend@example.com',
'from_name' => 'Michael',
'to' => array(
array(
'email' => 'example@gmail.com',
'name' => 'Lucas',
'type' => 'to'
)
),
'global_merge_vars' => array(
array(
'name' => 'NAME',
'content' => 'Lucas'
)
),
'merge_vars' => array(
array(
'rcpt' => 'example@gmail.com',
'vars' => array(
array(
'name' => 'NAME',
'content' => 'Lucas'
)
)
)
),
'attachments' => array(
array(
'type' => 'application/pdf',
'name' => 'test.pdf',
'content' => base64_encode(file_get_contents('test.pdf'))
)
),
'images' => array(
array(
'type' => 'image/png',
'name' => 'Smiley.png',
'content' => base64_encode(file_get_contents('Smiley.png'))
)
),
'subaccount' => 'cust-123'
);
try {
/* Отправка обычного письма */
$result = $mail->messages->send($data);
print_r($result);
/* Отправка письма с использованием шаблона */
$result = $mail->messages->sendTemplate('example', $tpl_con, $data);
print_r($result);
} catch(Mandrill_Error $error) {
echo 'Error: ' . get_class($error) . ' - ' . $error->getMessage();
}
The results of the two methods are presented below. The bottom image shows the result of applying the template.

We sent the same email using both methods available to us. In order to be able to see the differences. As an example, an image and a PDF document were attached to the letter.
Also, work with dynamic content and templates was demonstrated. The difference in using a template from dynamic content is that a label is specified for the template.
label writing format
* | name | * - the name is case-insensitive
This label will be replaced in the future by its content. And for dynamic content, the following attribute is specified in the HTML tag.
Making dynamic content
mc: edit = "section-name", the identifier is case sensitive
where, section-name is a unique identifier, which means that the data of the specified identifier will be inserted into the HTML tag containing it. It is also worth considering that the template elements specified in the global_merge_vars field (see table 1 ) will be replaced by the corresponding elements specified in the vars field of the merge_vars structure for the specified email addresses in the rcpt field of the merge_vars structure "
Consider the following example:
'to' => array(
array(
'email' => 'example_1@gmail.com',
'name' => 'Lucas 2',
'type' => 'to'
),
array(
'email' => 'example_2@gmail.com',
'name' => 'Lucas 1',
'type' => 'to'
)
),
'global_merge_vars' => array(
array(
'name' => 'NAME',
'content' => 'Lucas'
)
),
'merge_vars' => array(
array(
'rcpt' => 'example_1@gmail.com',
'vars' => array(
array(
'name' => 'NAME',
'content' => 'Alex'
)
)
)
)
Here the label will be redefined only for the recipient with the address “example_1@gmail.com”. Accordingly, the label in the template with the name “ NAME ” will be replaced with the name “ Alex ”, for the rest of the recipients the contents of the label will remain “ Lucas ”.
When using both methods to send letters, the following factors must be considered:
- When using the send () method :
- If the “html” field is indicated in the data structure (see Table 1 ) and it contains some data, then the “text” field will be blocked by it.
- When using the sendTemplate () method :
- If in the template editor (see above) the template has the “HTML” format, then none of the two fields: “html” and “text” will be able to overlap the text of the template;
- If in the template editor, the template has the “Text” format, then for its automatic substitution into the letter template when sending, it is forbidden to specify the fields: “html” and “text” in the data structure. You can specify it more correctly, but they should not contain any data, otherwise the data specified in one of the two fields in priority order (html, text) will go to the message body;
- If the “subject” field is not filled in the template, then the priority will go to the field of the same name (if any) in the data structure (see table 1 ).
The list of possible errors is contained in the table. 4.
Table 4 . The list of possible errors when sending a letter is unsuccessful
Invalid_Key | Invalid API key. |
Unknown_Message | Message ID does not exist. |
ValidationError | Parameters passed during the call are incorrect. |
Generalerror | An error occurred during the request. |