
Zendframework + ffmpeg + gearman + amazon = Video encoder service
I want to share with the habrasociety the experience of creating a web service for converting video and saving in cloud storage. Immediately make a reservation, the service is written for internal use by one European company, and has been operating for more than 6 months. One of the areas of the company is WebTv product. It was very problematic to deploy a structure for video conversion on each new site, and often these processes greatly impede the server.
It was decided to create a service that would satisfy the following requirements:
The most used scheme is POST video upload. On the client site, a flash loader is embedded in the form for adding a new video, which sends the file immediately to the video service along with the authorization data. The diagram below details all the steps:
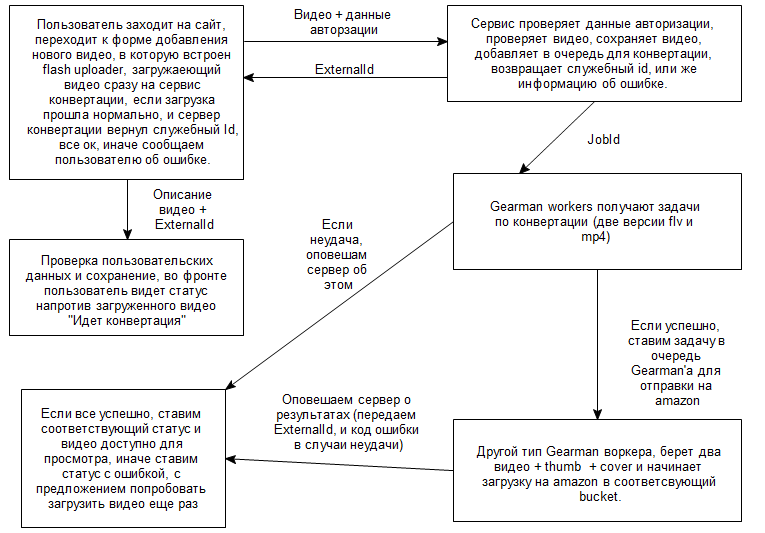
Authentication
Since login and password for authorization are available in html code, and this is not good, you had to come up with something. The solution was to use one-time passwords with a limited lifetime and a two-step authentication system. The client service generates a password and saves it to its database (as well as the generation time and the token is used / not used). After receiving a request, the video service knocks on the client server at a predetermined URL and receives a confirmation of the relevance of the password.
Checking the video
Naturally, we cannot blindly believe that the downloaded file is really a video file.
Using the ffmpeg_movie class, we try to find out information about the video file, if we were not able to return an error to the client server that the file cannot be used.
Actually, the task was to start converting the video after saving the request to the database. Naturally, the good old cron fits poorly here, launching once a minute, sequential execution. Before that I already used Gearman and my choice fell on this tool. In fact, I had to tinker with him, for some reason at first I did not want to work point-blank:
Workers constantly returned -1 and died. I remembered that in previous projects I did work a little differently. And he inherited from GearmanWorker, made the base layer for all types of worker:
and then I already collected the necessary workers:
Next, another unpleasant thing came out, the connection to the DB closed after some period of time, so I decided that I would close the connection before starting the conversion process:
Statistics show that it is enough to drive 3-4 workers to normally serve up to 10 requests per hour. Read more about Gearman here Gearman.org
With this “fruit” I have many sleepless nights. In the beginning, everything was trivial, I already had standard requests debugged, like to make resize, bit rates and all that - everything is trivial here. The most interesting thing started when it was necessary to resize and rotate and add the broadcast mask and paddings in one pass, in general, all this instead of no one wanted to work. Vfilters came to the rescue , which were added relatively recently. All the charm is that you can consistently apply filters, i.e. for example, first rotate, resize, add padding, hang watermarks. Here is what a typical ffmpeg command looks like in my service:
Because Since each request requires the creation of two flv + mp4 videos, at the end of the work, the data is synchronized and the worker, whose work was completed later, puts in line the task of downloading the finished data to amazon. If there are errors during the conversion, notify the server about this.
Another type of Gearman worker is uploading finished data to Amazon S3 using the standard Zend library for working with Amazon api.
In general, I want to say that over the past year, Amazon has upset several times, for example, a manager from Europe writes to me that he doesn’t play the video in the front, and I write to him, like this, I’m sitting right there watching this video that you’re watching does not work. Then it came to pass, we turned to various Amazon data centers. And there are also problems downloading large videos, about once a month, some videos may not load, and the Amazon server will return some kind of inaudible error code.
You can read more about Amazon S3 here at Amazon Simple Storage .
The service on demand can hang watermarks on the video, can rotate the video, correctly resize according to the specified parameters, filling the empty space with black. To do this, use the vfilters that come with ffmpeg in recent versions.
It is able to collect video from ftp, imap, amazon s3 storage and also convert it, then notifying the server (But these methods are used extremely rarely).
Actually a curious thing about IMAP, we are talking about collecting videos sent via MMS. I didn’t go into the implementation details; my business was simply to get into the mail using the IMAP protocol to find new messages, collect all the video files from them and process them.
And now the company is thinking about another goodies. Screw Asteriks, and for example, a client makes a video call from his phone, which supports 3G, leaves a video message, through Asteriks this thing gets into a special daddy. Every 5 minutes, a video service in ftp visits it if it finds something new, processes it, and returns to the client’s server a video ready for use.
For the last 3 months, the server has been working stably, and has never crashed. For half a year, more than 4000 requests were processed.
It is interesting to hear opinions, comments, constructive criticism.
It was decided to create a service that would satisfy the following requirements:
- Easy integration with any site.
- Implement the process of converting more than one video file at the same time.
- Implement the process of collecting video from ftp, IMAP and simply directly receive the video file via HTTP POST.
- Optionally, with the help of additional parameters, be able to rotate video, broadcast watermarks, etc.
- Implement a secure authorization system in the front, where users can see what data goes to the video service in addition to the video file.
- Send finished results to Amazon S3.
- Handle errors well, and notify client service about it.
The simplest scheme of integration with the service.
The most used scheme is POST video upload. On the client site, a flash loader is embedded in the form for adding a new video, which sends the file immediately to the video service along with the authorization data. The diagram below details all the steps:
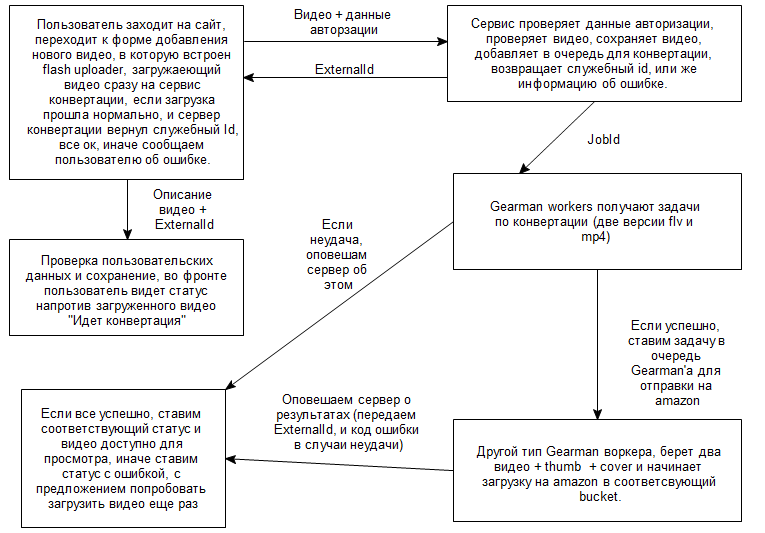
Checking data and video on the service side.
Authentication
Since login and password for authorization are available in html code, and this is not good, you had to come up with something. The solution was to use one-time passwords with a limited lifetime and a two-step authentication system. The client service generates a password and saves it to its database (as well as the generation time and the token is used / not used). After receiving a request, the video service knocks on the client server at a predetermined URL and receives a confirmation of the relevance of the password.
Checking the video
Naturally, we cannot blindly believe that the downloaded file is really a video file.
Using the ffmpeg_movie class, we try to find out information about the video file, if we were not able to return an error to the client server that the file cannot be used.
Gearman
Actually, the task was to start converting the video after saving the request to the database. Naturally, the good old cron fits poorly here, launching once a minute, sequential execution. Before that I already used Gearman and my choice fell on this tool. In fact, I had to tinker with him, for some reason at first I did not want to work point-blank:
function converter()
{
//Здесь код конвертера
}
$worker = new GearmanWorker();
$worker->addFunction("convert", "converter");
//и т.д.
Workers constantly returned -1 and died. I remembered that in previous projects I did work a little differently. And he inherited from GearmanWorker, made the base layer for all types of worker:
class App_Model_Worker_Abstract extends GearmanWorker
{
public function __construct($gearman)
{
parent::__construct();
if (!$this->addServer($gearman['host'], $gearman['port'])) {
throw new Exception("Can't connect to Gearman");
}
}
public function work()
{
while (parent::work());
return true;
}
}
and then I already collected the necessary workers:
// Конвертор
class App_Model_Worker_Converter extends App_Model_Worker_Abstract
{
public function __construct($gearman)
{
parent::__construct($gearman);
//Тут еще немного кода
}
public function action($job)
{
$fileId = $job->workload();
$file = $this->_modelFileResponse->getPrepareToConvertFile($fileId);
//Собственно здесь конвертируем.
}
}
//Воркер, который отправляет готовые результаты в хранилище
class App_Model_Worker_SendResponse extends App_Model_Worker_Abstract
{
public function __construct($gearman)
{
parent::__construct($gearman);
}
public function action($job)
{
$responseId = $job->workload();
//Тут собственно код загрузчика.
}
}
Next, another unpleasant thing came out, the connection to the DB closed after some period of time, so I decided that I would close the connection before starting the conversion process:
$model->getAdapter()->closeConnection();
Statistics show that it is enough to drive 3-4 workers to normally serve up to 10 requests per hour. Read more about Gearman here Gearman.org
Ffmpeg
With this “fruit” I have many sleepless nights. In the beginning, everything was trivial, I already had standard requests debugged, like to make resize, bit rates and all that - everything is trivial here. The most interesting thing started when it was necessary to resize and rotate and add the broadcast mask and paddings in one pass, in general, all this instead of no one wanted to work. Vfilters came to the rescue , which were added relatively recently. All the charm is that you can consistently apply filters, i.e. for example, first rotate, resize, add padding, hang watermarks. Here is what a typical ffmpeg command looks like in my service:
/usr/local/bin/ffmpeg -i "/home/encoderws/public_html/data/files/requests/example-test-5c2a1c8a2e9e540690ba05670a526872_d917a8705cade8efdd87008df20ef19c" -acodec libfaac -vcodec mpeg4 -b 400k -ar 44100 -f mp4 -ab 96k -ac 2 -vf "[in] rotate=90 [rt0], [rt0] scale=203:360 [sc0], [sc0] pad=640:360:219 [pd0], [pd0] scale=640:360 [sc1], movie=0:png:/home/encoderws/public_html/data/files/watermarks/watermark.png [wm], [sc1] [wm] overlay=0:0:1 [out]" -y '/home/encoderws/public_html/data/files/responses/example-test-5c2a1c8a2e9e540690ba05670a526872_d917a8705cade8efdd87008df20ef19c.mp4
Because Since each request requires the creation of two flv + mp4 videos, at the end of the work, the data is synchronized and the worker, whose work was completed later, puts in line the task of downloading the finished data to amazon. If there are errors during the conversion, notify the server about this.
Amazon
Another type of Gearman worker is uploading finished data to Amazon S3 using the standard Zend library for working with Amazon api.
In general, I want to say that over the past year, Amazon has upset several times, for example, a manager from Europe writes to me that he doesn’t play the video in the front, and I write to him, like this, I’m sitting right there watching this video that you’re watching does not work. Then it came to pass, we turned to various Amazon data centers. And there are also problems downloading large videos, about once a month, some videos may not load, and the Amazon server will return some kind of inaudible error code.
You can read more about Amazon S3 here at Amazon Simple Storage .
Other goodies
The service on demand can hang watermarks on the video, can rotate the video, correctly resize according to the specified parameters, filling the empty space with black. To do this, use the vfilters that come with ffmpeg in recent versions.
It is able to collect video from ftp, imap, amazon s3 storage and also convert it, then notifying the server (But these methods are used extremely rarely).
Actually a curious thing about IMAP, we are talking about collecting videos sent via MMS. I didn’t go into the implementation details; my business was simply to get into the mail using the IMAP protocol to find new messages, collect all the video files from them and process them.
And now the company is thinking about another goodies. Screw Asteriks, and for example, a client makes a video call from his phone, which supports 3G, leaves a video message, through Asteriks this thing gets into a special daddy. Every 5 minutes, a video service in ftp visits it if it finds something new, processes it, and returns to the client’s server a video ready for use.
For the last 3 months, the server has been working stably, and has never crashed. For half a year, more than 4000 requests were processed.
It is interesting to hear opinions, comments, constructive criticism.