React tutorial, part 10: workshop on working with component properties and styling
- Transfer
- Tutorial
Today, in the tenth part of the translation of the training course on React, we suggest you complete a practical task of working with the properties of components and their styling.
→ Part 1: course overview, reasons for the popularity of React, ReactDOM and JSX
→ Part 2: functional components
→ Part 3: component files, project structure
→ Part 4: parent and child components
→ Part 5: starting work on a TODO application, basics of styling
→ Part 6: about some features of the course, JSX and JavaScript
→ Part 7: inline styles
→ Part 8: continued work on a TODO application, familiarity with the properties of components
→
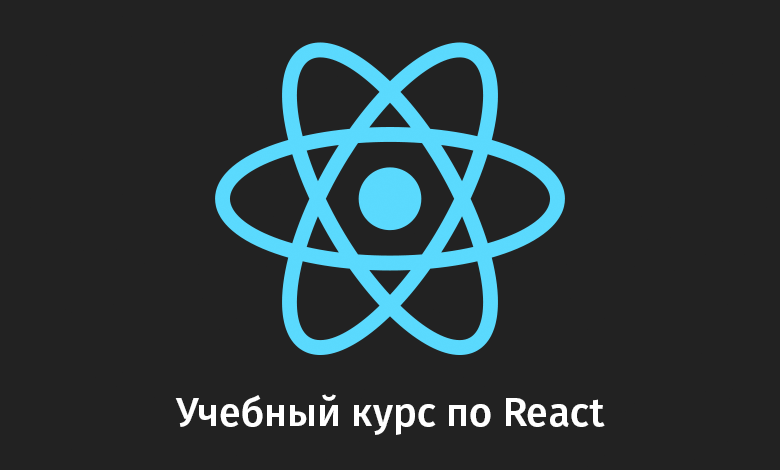
Part 9: properties of components
→ Part 10: workshop on working with properties of components and stylization
→ Part 11: dynamic markup generation and the array method map
→ Part 12: workshop, third stage of working on a TODO application
→ Part 13: class-based components
→ Part 14: a workshop on class-based components, state of components
→ Part 15: workshops on working with the state of components
→ Part 16: fourth stage of working on a TODO application, event handling
→ Part 17: fifth stage of working on a TODO application, modification status Components
→Part 18: the sixth stage of working on a TODO application
→ Part 19: methods of life cycle of components
→ Part 20: the first lesson on conditional rendering
→ Part 21: the second lesson and practice on conditional rendering
→ Part 22: the seventh stage of working on a TODO application, loading data from external sources
→ Part 23: first lesson on working with forms
→ Part 24: second lesson on working with forms
→ Part 25: practical work on working with forms
→ Original
Some jokes consist entirely of a key phrase. For example: "It's hard to explain punishment to kleptomaniacs because they always take things literally." Think about how a component
The file
Here is the file code
Note that since the component file
Here is the file code
Here, at function declaration
From the component, we return several elements - so they are enclosed in a tag
This is what the application project in VSCode looks like.

Application in VSCode
Here is the application page.

Application page in the browser
Recall that the main purpose of the additional task is to organize the correct conclusion of anecdotes, which consist entirely of a key phrase. This is expressed in the fact that, when creating an instance of a component
If you put this code in the upper part of the code returned by the component

Incorrectly formed application page.
Obviously, the problem here is that, although the property is not transferred to the component
Looking ahead, we’ll note that in future parts of the course we’ll talk about conditional rendering. With this approach to rendering, you can effectively solve problems like ours. In the meantime, we will try to use the page styling tools. Namely, we will make it so that if the property is not transferred to the component
Here, the style
Now the application page in the browser will look like below.

Correct processing by a component of a situation in which the question property is not passed to it.
You may notice that all the elements formed by the component
But what looks like what is now displayed on the application page.

Styling an element that is different from others.
Now that we have worked on a component
In this practical lesson, we were working on the skills of transferring properties to components. If you analyze the code of the example given here, you will notice that in order to output several similar markup blocks, you have to constantly write repetitive JSX code fragments. In the next lesson, we will talk about how to use automated JavaScript tools to automate the formation of such pages, at the same time separating the markup and data.
Dear readers! If your solution to the tasks of this workshop differs from the proposed one, we ask you to tell about it.

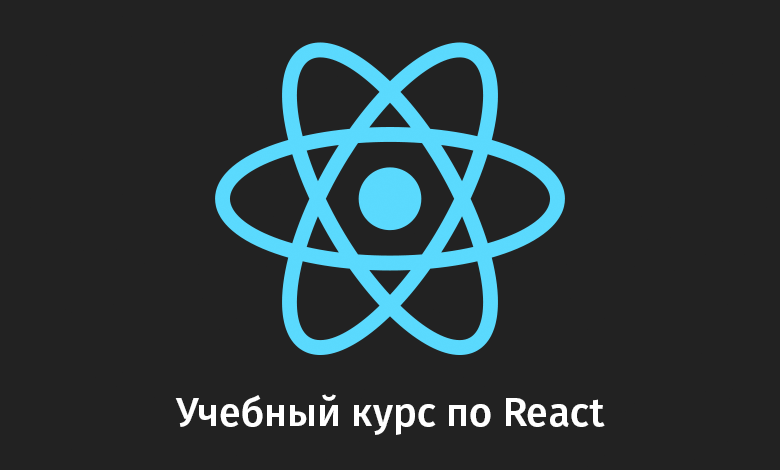
Part 9: properties of components
→ Part 10: workshop on working with properties of components and stylization
→ Part 11: dynamic markup generation and the array method map
→ Part 12: workshop, third stage of working on a TODO application
→ Part 13: class-based components
→ Part 14: a workshop on class-based components, state of components
→ Part 15: workshops on working with the state of components
→ Part 16: fourth stage of working on a TODO application, event handling
→ Part 17: fifth stage of working on a TODO application, modification status Components
→Part 18: the sixth stage of working on a TODO application
→ Part 19: methods of life cycle of components
→ Part 20: the first lesson on conditional rendering
→ Part 21: the second lesson and practice on conditional rendering
→ Part 22: the seventh stage of working on a TODO application, loading data from external sources
→ Part 23: first lesson on working with forms
→ Part 24: second lesson on working with forms
→ Part 25: practical work on working with forms
Lesson 20. Workshop. Component properties, styling
→ Original
▍Job
- Create a new React application project.
- Print on the application page the component
App
whose code should be in a separate file. - Component App should display 5 components
Joke
containing jokes. Print these components as you like. - Each component
Joke
must accept and process the propertyquestion
, for the main part of the anecdote, and the propertypunchLine
for its key phrase.
▍Advanced task
Some jokes consist entirely of a key phrase. For example: "It's hard to explain punishment to kleptomaniacs because they always take things literally." Think about how a component
Joke
can only display a property passed to punchLine
it, if the property is question
not set. Experiment with styling components.▍Decision
Main task
The file
index.js
will look quite familiar:import React from"react"import ReactDOM from"react-dom"import App from"./App"
ReactDOM.render(<App />,
document.getElementById("root"))
Here is the file code
App.js
:import React from"react"import Joke from"./Joke"functionApp() {
return (
<div>
<Joke
question="What's the best thing about Switzerland?"
punchLine="I don't know, but the flag is a big plus!"
/>
<Joke
question="Did you hear about the mathematician who's afraid of negative numbers?"
punchLine="He'll stop at nothing to avoid them!"
/>
<Joke
question="Hear about the new restaurant called Karma?"
punchLine="There’s no menu: You get what you deserve."
/>
<Joke
question="Did you hear about the actor who fell through the floorboards?"
punchLine="He was just going through a stage."
/>
<Joke
question="Did you hear about the claustrophobic astronaut?"
punchLine="He just needed a little space."
/>
</div>
)
}
export default App
Note that since the component file
Joke
is located in the same folder as the component file App
, we import it with the command import Joke from "./Joke"
. From App
we return several elements, so the entire output needs to be wrapped in a certain tag, for example, in a tag <div>
. To component instances we transfer properties question
and punchLine
. Here is the file code
Joke.js
:import React from"react"functionJoke(props) {
return (
<div>
<h3>Question: {props.question}</h3>
<h3>Answer: {props.punchLine}</h3>
<hr/>
</div>
)
}
exportdefault Joke
Here, at function declaration
Joke
, we specify the parameter props
. Recall that this name is used according to the established tradition. In fact, it can be any, but it is better to call it that props
. From the component, we return several elements - so they are enclosed in a tag
<div>
. With the help of constructions props.question
, props.punchLine
we refer to the properties passed to the component instance when it is created. These properties become properties of the object props
. They are enclosed in braces due to the fact that the JavaScript code used in the JSX markup needs to be in curly braces. Otherwise, the system will accept variable names as plain text. After a couple of items<h3>
, in one of which the main text of the anecdote is displayed, and in the other - its key phrase, there is an element <hr/>
describing a horizontal line. These lines will be displayed after each joke, separating them. This is what the application project in VSCode looks like.

Application in VSCode
Here is the application page.

Application page in the browser
Additional task
Recall that the main purpose of the additional task is to organize the correct conclusion of anecdotes, which consist entirely of a key phrase. This is expressed in the fact that, when creating an instance of a component
Joke
, only a property is passed to it punchLine
, but the property is question
not transferred. Creating an instance of such a component looks like this:<Joke
punchLine="It’s hard to explain puns to kleptomaniacs because they always take things literally."
/>
If you put this code in the upper part of the code returned by the component
App
, the application page will take the following form.
Incorrectly formed application page.
Obviously, the problem here is that, although the property is not transferred to the component
question
, it displays text that precedes the main part of each anecdote, after which nothing is output. Looking ahead, we’ll note that in future parts of the course we’ll talk about conditional rendering. With this approach to rendering, you can effectively solve problems like ours. In the meantime, we will try to use the page styling tools. Namely, we will make it so that if the property is not transferred to the component
question
, the corresponding fragment of the JSX markup returned by it would not be displayed on the page. Here is the complete component codeJoke
, which implements one of the possible approaches to solving our problem with CSS:import React from"react"functionJoke(props) {
return (
<div>
<h3style={{display:props.question ? "block" : "none"}}>Question: {props.question}</h3>
<h3>Answer: {props.punchLine}</h3>
<hr/>
</div>
)
}
exportdefault Joke
<h3>
We assign the
first element a style that is defined in the process of creating a component instance based on the presence of a property in the object props.question
. If this property is in the object, the element accepts the style display: block
and is displayed on the page, if not, it is display: none
not displayed on the page. In addition, the use of this design will result in:<h3 style={{display: !props.question && "none"}}>Question: {props.question}</h3>
Here, the style
display: none
is assigned to the element if the object props
does not have a property question
, otherwise display
nothing is assigned to the property . Now the application page in the browser will look like below.

Correct processing by a component of a situation in which the question property is not passed to it.
You may notice that all the elements formed by the component
Joke
look the same. We will think about how to select those of them that only the property is passed topunchLine
. We solve this problem using the built-in styles, and the approach that we considered above. Here is the updated component codeJoke
:import React from"react"functionJoke(props) {
return (
<div>
<h3style={{display: !props.question && "none"}}>Question: {props.question}</h3>
<h3style={{color: !props.question && "#888888"}}>Answer: {props.punchLine}</h3>
<hr/>
</div>
)
}
exportdefault Joke
But what looks like what is now displayed on the application page.

Styling an element that is different from others.
Now that we have worked on a component
Joke
, it has become more versatile and better suited for reuse.Results
In this practical lesson, we were working on the skills of transferring properties to components. If you analyze the code of the example given here, you will notice that in order to output several similar markup blocks, you have to constantly write repetitive JSX code fragments. In the next lesson, we will talk about how to use automated JavaScript tools to automate the formation of such pages, at the same time separating the markup and data.
Dear readers! If your solution to the tasks of this workshop differs from the proposed one, we ask you to tell about it.
