
Unity: Particle Systems
- Transfer
- Tutorial

Particle systems are like salt: even a small amount can add style to the upcoming “dish”. Modern games that do not use particle systems look rather pale.
In the past, we had to program each wisp of smoke on our own. Fortunately, with its built-in modular particle system, Unity Shuriken makes particle creation a fairly simple task. This particle system is easy to learn and allows you to create complex effects.
In this tutorial you will learn the following:
- How to add new particle systems to Unity.
- You will learn which modules of particle systems are used most often and learn how to use them.
This tutorial consists of two main parts: in the first we will create a torch flame, in the second - the effect of a bomb explosion.
Note: this tutorial assumes that you are familiar with the basics of working with Unity. If you are new to Unity or need to brush up on your knowledge, first check out our Introduction to Unity tutorial .
Getting started with particle systems
Download the draft for this tutorial and unzip it to a place convenient for you. Please note that to use the tutorial you will need at least Unity 2017.2.
Open the Starter Project in Unity. Assets inside it are distributed in several folders:
- Materials : contains fire material.
- Models : Contains torch and bomb models, as well as their materials.
- Prefabs : contains prefab bombs.
- Scenes : Contains Torch and Bomb scenes.
- Scripts : here are the source scripts.
- Textures : here is the texture for the fire material.
Having figured out where what is located, we can begin to study the operation of particle systems in Unity.
Adding a particle system
In the general case, a system of particles emits particles at random points within a predetermined space, which may take the form of, for example, a sphere or cone. The system determines the lifetime of the particle itself, and when it ends, the system destroys the particle.
Particle systems are convenient in that they are components that can be added to any GameObject in the scene. Want to get laser beams out of the eyes of sharks? Just add a particle system to GameObject's shark eyes!
Open the Torch scene in the Project Window and run the scene:

So far, nothing interesting is happening here. The torch hangs on the wall, but no fire is visible on it. First we need to add a particle system.
Stop the scene and select TorchFireParticles in the Hierarchy. In the Inspector, click on the Add Component button . Find the Particle System and click to add it:

Note: You may have noticed that I did not add the particle system directly to GameObject MedievalTorch. I made the particles emit from the tank with fuel in the upper part, and not from the middle of the torch.
Run the scene and you will see that the particles are already emitting:
GIF

Note: you can see pink particles instead of white. This seems to be a Unity bug with setting the default texture. If this happened to you, then do not worry, soon we will set the correct flame texture. If you want to fix this bug now, click on the Renderer section of the particle system, click on the point next to the Material field and double-click Default-Particle in the window that opens .
When you select a GameObject with a particle system attached to it, you will notice a black dialog box in the lower right corner of the scene window. This dialog box allows you to simulate or stop a particle system. Pressing the Simulate button activates the particle system and replaces it with the “Pause" button. To stop the simulation, click on the “Stop” button.
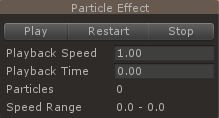
This dialog box is useful when creating particle systems that run for a limited period of time, such as for explosions.
We consider the particle system more closely.
Take a look at the Inspector. You will notice that the particle system component added by us has several subsections:
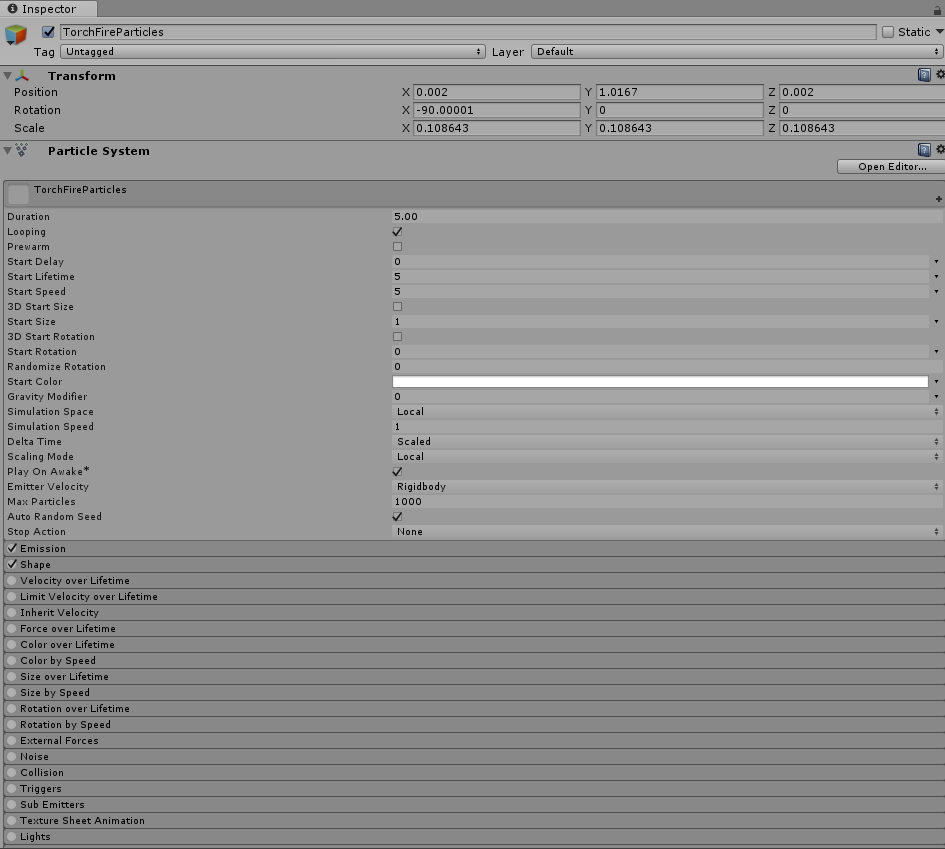
Each of these subsections is called a Module . These modules contain particle system parameters. The module deployed by default is called the Main module :
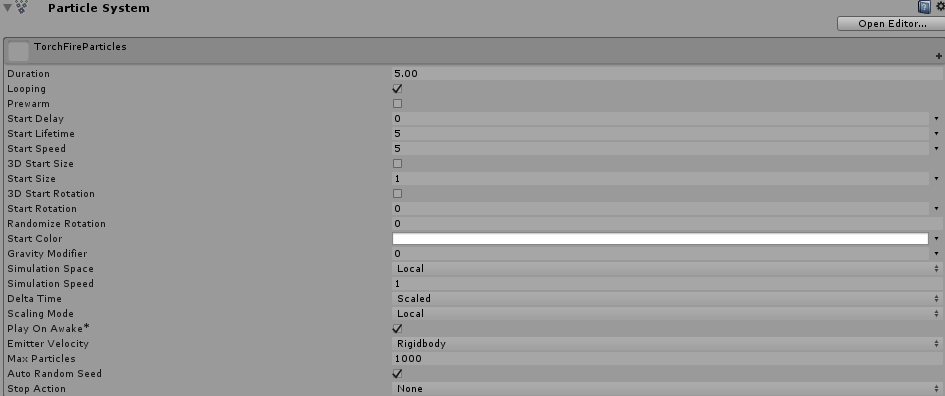
The Main module is the foundation of any particle system in Unity. Here are the most basic particle parameters:
- Duration : particle system runtime in seconds. We leave here the default value of 5.00 .
- Looping : re-emitting particles until the particle system stops. The cycle restarts after reaching the Duration time . The fire should be on continuously, so leave this option on.
Enabled and Disabled - Prewarm : Used only when Looping is enabled . The particle system will behave as if it had completed a full cycle at startup.GIF
On and off
For the fire effect, turn it off so that it seems that the torch has just been set on fire. - Start Delay : The delay in seconds before the particle system begins to emit particles. Leave here the default value of 0 .
- Start Lifetime : The initial particle lifetime in seconds. After the completion of time, the particle is destroyed.
Top: 8 seconds. Below: 3 seconds
Let us make the lifetime equal to 4 seconds; thanks to this, the flame will not be too high. - Start Speed : The initial particle speed. The greater the speed of the particles, the more they will propagate.
Above: 2. Bottom: 10
Set the speed value to 0.75 ; so the flame effect will be slower and denser.
Note: changing the parameters of the particle system, you will see a preliminary overview of it in the Game window. Take a look at this preliminary review as you complete the tutorial.
Run the scene to see how the changes made affect:
GIF

We set up the torch, but the effect of the fire so far leaves much to be desired. Fortunately, the Main module has additional options to improve the shape and behavior of the torch.
Other properties of the Main module
In the hierarchy, select GameObject TorchFireParticles and scroll down to the particle system. In the Main Module, look at the following properties:
- Start Size : The initial particle size.
Top: 0.2. Bottom: 1.
Choose size 3 ; This is a convenient size that allows you to more clearly see individual particles. - Start Rotation : The initial angle of rotation of the particles.
Top: 0 °. Bottom: 45 °
Leave the value 0 ° ; the particles are round, so you are unlikely to notice the difference. - Start Color : The initial color of the particles. Leave the color equal to the original pure white (255,255,255) ; we will color the fire using texture.
- Gravity Modifier : Scales the gravity value specified in the Unity Physics Manager window . If it is 0, then gravity will be disabled.GIF
This is an image from a system with a gravity modifier equal to 1 , that is, particles fall down like a waterfall. Make gravity in your system equal to 0 ; particles will move up at the speed specified in Start Speed . - Simulation Space : Moves particles to Local Space with a particle system. When World Space is selected, particles move freely after emission.
Top: local space. Bottom: world space
Leave the value Local Space for your simulation . The influence of the parameter will be noticeable only when the system of particles moves. - Play On Awake : Starts to emit particles immediately after turning on. If this option is disabled, then you will have to manually start the particle system through a script or animation system. Leave it on because we want the flame to start burning when the scene starts.
- Max Particles : The maximum number of particles that can be alive in a particle system at a time. If you try to emit more particles than the value set here, then they will not be emitted at all. This parameter is mainly needed for performance reasons, and the default value of 1000 particles in our case is more than enough.
Wow, that’s the list! But as a result, we learned how to add a particle system to the scene and customize it to your liking.
Run the scene again to see how the changes affected it:
GIF

Each time it becomes more and more like a flame, right?
However, we need more particles. To do this, we need to change the emission system.
Introducing the Emission Module
The Emission module controls the number and time of emitted particles in the system and allows you to create any effects, from a constant flow to a sharp explosion of particles.
From the Particle System Inspector, click on the title of the Emission module:

This will open the Emission module:

Rate over Time represents the number of particles emitted per second. Set Rate over Time to 15 .
Run the scene again; now the particle system will look more like a burning flame.
GIF

But it is noticeable that it still looks more like smoke. And here we will make the biggest change at the current stage of the tutorial: our own texture!
Adding your own texture
All particles have a particle material and a texture that defines their appearance. With the default texture, we can do just that. Replacing the texture, we can create effects like magic stars, smoke and, of course, fire.
Replacing the particle texture is quite simple. Until this moment, particles were drawn on the screen using the Default-Particle material, which is a material of particles with a circular gradient texture:

To replace this material, select TorchFireParticles in the GameObject hierarchy . Then, find the particle system component in the inspector and open the particle system Renderer module .
Open the Materials folder in the Project View and drag it onto the FireMaterial Material onto the Material property :
GIF

Then run the scene to see how our selected texture is applied:
GIF

Feel the heat? However, the flame is too wide; To fix this, we need to change the shape of the particle system.
Particle system shape change
The Shape module , as the name implies, controls the shape and behavior of particles in this shape. You can choose from several different forms; each has its own parameters. This allows you to create particles in the box, in a sphere, or even in your own mesh!
Expand the Shape module in the inspector:

As the shape ( shape ) a system of particles selected cone ( cone ), i.e. particles are emitted from the bottom base and moving outward at an angle ( angle ):

In the example shown above, the base is colored blue , the angle is green , and the particles are red . It is also worth noting that when the Shape module is expanded, we see a convenient preview of the cone in the Scene window:

By changing Angle , you can resize the cone, making it wider or narrower. Specify a value of 7 ; so we get a convenient narrow border that expands a bit when the particles rise up.
By changing Radius , we can change the size of the base. The larger the value, the more particles will be scattered upon emission. Select a value of 0.2 ; in this way we will ensure the creation of a flame inside the tank for the fuel of the torch.
Launch the scene and see how the shape of the flame changes:
GIF

It begins to look like a real flame! The last thing you can do is change the size of the particles over time.
Resize over time
Using the Size over Lifetime module, you can create particles that increase or decrease over the course of their lives, or even pulsate, like fireflies in a forest.
Locate the Size over Lifetime section in the list of particle system modules . The default is off, so check the box next to the module name:

Expand the Size over Lifetime module by clicking on its name. So you open a dark gray background with a flat curve ( Curve ) at the top:

Click on the dark gray background to open the curve editor at the bottom of the inspector. The horizontal axis represents the particle lifetime, and the vertical axis represents its size:

To edit the curve, you can move key points on both sides of the red line; You can add new keys by double-clicking on any part of the curve. To delete key points, right-click on the point and select Delete Key . You can also select one of the previously created curves at the bottom of the window:
GIF

Remember how flame tongues behave in the real world: they decrease when particles rise up. To simulate this behavior, we will select the third finished curve on the right to create a smoothly descending curve:

Run the scene to see the effect in its entirety!
GIF

Congratulations! You learned how to set up a new particle system and modify it as you wish to create a beautiful fire effect.
In the next section, we wake up inside Michael Bay to learn how to create explosive effects!
Bomb effect
Creating an explosion effect in Unity is quite simple. Once you figure out how to create particle instances as you see fit, you can use this effect for things like car wheels that throw sparks when rubbing against the ground, or balloons that explode and throw confetti out of you.
Open the Bomb scene in the Project Window and run the scene:

There is sex at the bottom of the scene, but nothing else happens in the scene except for it.
To create bombs, transfer the Bomb prefab to the Bomb Emitter prefab slot :
GIF

Run the scene again to see the bombs appear:
GIF

The emitter creates a new bomb every two seconds. To rotate them , you need to add a small rotational force when creating the bomb.
Open the Bomb script in the Scripts folder of the Project Window.
Add to the
Start()
following code:void Start()
{
float randomX = UnityEngine.Random.Range (10f, 100f);
float randomY = UnityEngine.Random.Range (10f, 100f);
float randomZ = UnityEngine.Random.Range (10f, 100f);
Rigidbody bomb = GetComponent ();
bomb.AddTorque (randomX, randomY, randomZ);
}
The first three lines generate random float values from 10 to 100 for the x, y, and z axes. Next, we get a link to the Rigidbody component of the bomb and apply a moment to it. Thanks to this, the bomb begins to rotate in a random direction. Save the changes to the script, return to Unity and run the scene.
GIF

Now bombs rotate beautifully when dropped. But I promised you explosions!
In the hierarchy, click the Create button and select Create Empty . Click on the created GameObject and name it ExplosionParticles . Next, add a new particle system to this GameObject. If you forgot how to create a particle system, then see the first section of the tutorial.
Once you have created the particle system, drag GameObject ExplosionParticles from the hierarchy to the Prefabs folder in Project Browser. After that, remove GameObject ExplosionParticles from Project Hierarchy.
GIF

Then select the Bomb prefab in the Prefabs folder and drag the ExplosionParticles prefab into the Explosion Particles Prefab Bomb slot as follows:
GIF

Now, when a bomb touches the ground, a new GameObject Explosion Particles will be created.
Run the scene to see what the explosion looks like. If you again have a bug with pink textures, then do not worry, we will change the texture.
GIF

Very magical , but so far unlike an explosion!
As with the torch, we will use Fire material for the particle system.
Select the ExplosionParticles prefab in the Project Window, and then expand the Renderer module in the inspector . Drag FireMaterial from the Materials folder from the Project Window to the Material slot , as shown below:
GIF

To complete the effect, we need to change the following parameters in the Main module:

- Set Duration to 0.70 .
- Looping needs to be disabled. Particles should be emitted only once.
- Set Start Lifetime to 0.7 .
- Set Start Speed to 10 .
- Set Start Size to 2 .
- Set Gravity Modifier to 1 . Due to this, the particles at the end will slightly go down.
Launch the bomb scene to see. what we created:
GIF

Well, that already sounds like an explosion, but you can definitely do better!
Blast creation
To improve the explosion, we will change the properties of the modules of the particle system. You guess which module we will change? Here's a tip - we already used it.
If you decide that this is an Emission module, then you are great!
Expand Emission Module . Rate is the number of particles created per second. For an explosion, we need not a constant stream of particles, but a sharp flash.
Set Rate over Time to 0 . Now go to Rate over Distance and you will see a list of Bursts , which is empty by default:

Burst is a collection of particles emitted simultaneously at a given point in time.
Click on the + button in the lower right corner to add a new Burst. You will see two fields: Time and Count :

Leave Time to 0 , and set Count to 150 . Such parameters will cause the particle system to simultaneously emit 150 particles at the beginning of the system.
Run the scene. How does everything look now?
GIF

Now this is more like an explosion! Although this explosion looks better, it still has an ugly conical shape, and the particles do not fade, they just disappear. To give the explosion a final look, he needs to set the correct shape.
First, expand the Shape module :

We have already used this module for the flame shape of the torch, but here you can choose other forms. Click on the drop-down box that says Cone to see all available options:

Each form affects the emitter in its own way. Each animation shown below shows the same emitter, with only the shape changing:
Sphere

Hemisphere (HemiSphere)

Cone
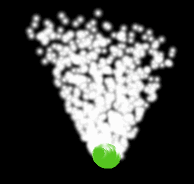
Box (Box)

Mesh (cube)

Circle

Edge

From one system you can get many different effects, just changing its shape! To create a realistic explosion, select the Sphere shape .
Launch the scene and get ready to explode :
GIF

Now everything looks amazing!
Although the explosion is beautiful, we have a small problem. Particles just disappear. This creates a feeling of trembling and looks completely unnatural. Instead of just disappearing, the particles should fade over time so that the explosion disappears gradually.
Color change
With the particle system open in the inspector, click on the checkbox next to the Color over Lifetime module to enable it, and then expand it. You will immediately see the word Color with a white block next to it. Click on the white block :

This opens the gradient editor:

The change in color over the lifetime of the particles is presented as a gradient. The initial color is on the left, and the particles gradually change color to the one indicated on the right:

Four white stripes at the edges are called markers ; Click between the two available markers to add a new one. To remove a marker, drag it from the strip:
GIF

Upper markers control Alpha or color opacity, and lower markers control RGB color values (Red, Green, Blue).

Click on the rightmost alpha marker. The current alpha value will be shown at the bottom of the gradient editor:

Drag the slider to 0 . Now the particles will gradually become transparent over the course of their lifetime.
Run the scene again to see how the changes made affect:
GIF

A wonderful explosion!
Want to make scenes even better? Go back to the torch scene and make the flame use the Size Over Lifetime Module to get a similar effect.
Where to go next?
The finished project can be downloaded from here .
In this tutorial, you learned how particle systems and their modules work in Unity, and also learned how to configure them to get the desired effect. Experiment with various parameters to see what other effects you can achieve.
For more information on the Shuriken particle system and its modules, see the official Unity documentation and the video on particle systems . You can also learn more about particle system scripting here .